


How Can Pandas' `melt()` Function Restructure Tabular Data from Columns to Rows?
Restructure Tabular Data with Pandas: Converting Columns to Rows
Dealing with data organized in columns for different dates or categories can be cumbersome. Pandas provides a powerful solution to convert such columns into easily readable rows. This article addresses the specific challenge of transforming a CSV with location information and multiple date columns into a desired format.
Problem Statement:
Consider a dataset with information organized by location, each with multiple dates as column headers. The goal is to restructure the data into a format where each row represents a unique location, name, date, and its corresponding value.
Pandas Solution:
Pandas offers a highly efficient method to achieve this transformation using its melt function.
Code:
df.melt(id_vars=["location", "name"], var_name="Date", value_name="Value")
Explanation:
- id_vars: Specifies the columns that remain unchanged as rows.
- var_name: Assigns a new column name for the melted dates.
- value_name: Provides a name for the column containing the original values.
Result:
Applying the melt function to the provided dataset yields the desired output:
location | name | Date | Value |
---|---|---|---|
A | "test" | Jan-2010 | 12 |
B | "foo" | Jan-2010 | 18 |
A | "test" | Feb-2010 | 20 |
B | "foo" | Feb-2010 | 20 |
A | "test" | March-2010 | 30 |
B | "foo" | March-2010 | 25 |
This new arrangement makes it easier to analyze the data by location, name, and date.
Note:
For older versions of Pandas (0.20 or earlier), the following alternate approach can be used:
df2 = pd.melt(df, id_vars=["location", "name"], var_name="Date", value_name="Value") df2 = df2.sort(["location", "name"])
The above is the detailed content of How Can Pandas' `melt()` Function Restructure Tabular Data from Columns to Rows?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




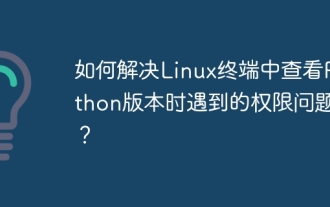
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
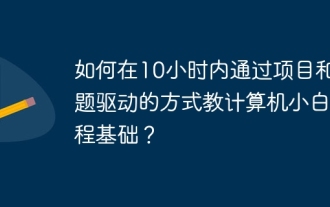
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
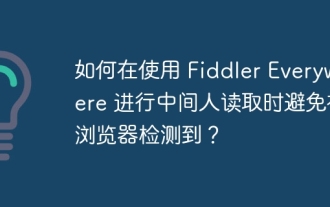
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
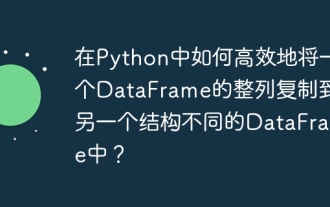
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
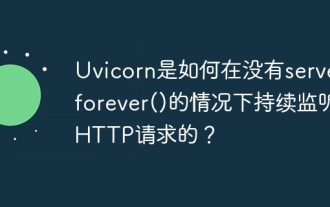
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
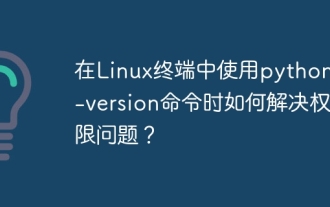
Using python in Linux terminal...
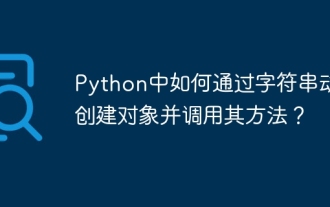
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
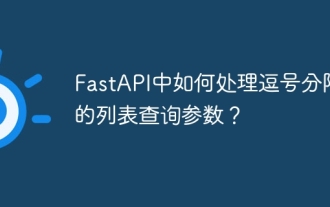
Fastapi ...
