How to Unnest List Columns in Pandas DataFrames?
Unnesting Columns with Pandas
When dealing with DataFrames that contain columns of lists, it can be useful to "unfold" these lists into separate rows.
Method 1: Using DataFrame.explode() (Pandas >= 0.25)
For single-column explosion, use explode() directly:
df = pd.DataFrame({'A': [1, 2], 'B': [[1, 2], [1, 2]]}) df_exploded = df.explode('B')
Method 2: Apply Series
df_exploded = df.set_index('A').B.apply(pd.Series).stack().reset_index(level=0).rename(columns={0:'B'})
Method 3: Repeat DataFrame
df_exploded = pd.DataFrame({'A':df.A.repeat(df.B.str.len()),'B':np.concatenate(df.B.values)})
Method 4: Reindex/Loc
df_exploded = df.reindex(df.index.repeat(df.B.str.len())).assign(B=np.concatenate(df.B.values))
Method 5: ChainMap
from collections import ChainMap d = dict(ChainMap(*map(dict.fromkeys, df['B'], df['A']))) df_exploded = pd.DataFrame(list(d.items()),columns=df.columns[::-1])
Method 6: Numpy
newvalues=np.dstack((np.repeat(df.A.values,list(map(len,df.B.values))),np.concatenate(df.B.values))) df_exploded = pd.DataFrame(data=newvalues[0],columns=df.columns)
Method 7: Iterators
from itertools import cycle,chain l=df.values.tolist() l1=[list(zip([x[0]], cycle(x[1])) if len([x[0]]) > len(x[1]) else list(zip(cycle([x[0]]), x[1]))) for x in l] df_exploded = pd.DataFrame(list(chain.from_iterable(l1)),columns=df.columns)
Generalization to Multiple Columns
To generalize the above methods for multiple columns, use the following function:
def unnesting(df, explode): idx = df.index.repeat(df[explode[0]].str.len()) df1 = pd.concat([ pd.DataFrame({x: np.concatenate(df[x].values)}) for x in explode], axis=1) df1.index = idx return df1.join(df.drop(explode, 1), how='left')
Column-Wise Unnesting
To unnest horizontally, modify the function:
def unnesting(df, explode, axis): if axis==1: # Previous implementation else : df1 = pd.concat([ pd.DataFrame(df[x].tolist(), index=df.index).add_prefix(x) for x in explode], axis=1) return df1.join(df.drop(explode, 1), how='left')
The above is the detailed content of How to Unnest List Columns in Pandas DataFrames?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
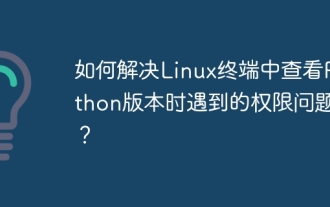
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
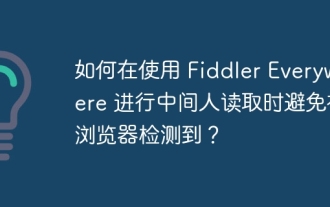
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
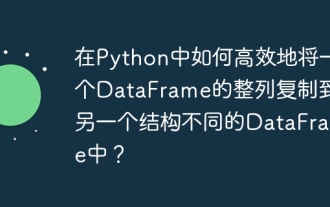
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
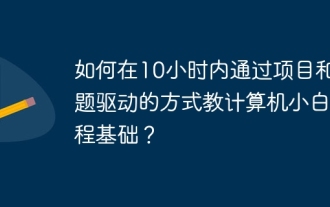
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
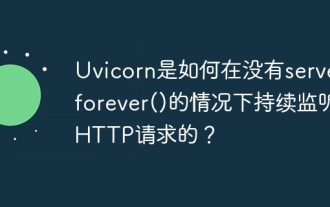
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
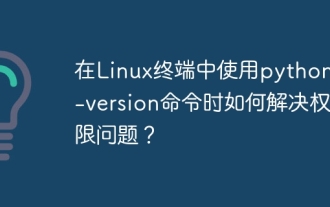
Using python in Linux terminal...
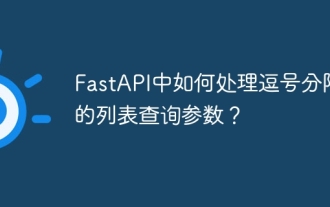
Fastapi ...
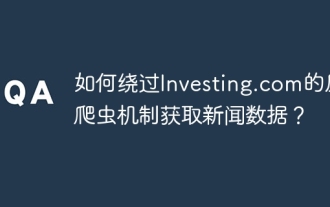
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
