How to Convert Go's time.Time Objects to Strings for String Arrays?
Converting time.Time Objects to Strings in Go
In Go, converting time.Time objects to strings is essential when working with timestamp data, arrays, or displaying time information in a human-readable format. This article addresses a common issue that arises when attempting to add time.Time values to []string arrays, and provides a solution to convert these values to strings for successful array construction.
The Issue:
When trying to add a time.Time value to a []string array, you may encounter the following error:
cannot use U.Created_date (type time.Time) as type string in array element
This error indicates that time.Time values, representing timestamps, cannot be directly added to string arrays.
The Solution:
To resolve this issue, Go provides the String() and Format() methods for time.Time objects. These methods allow you to convert timestamps into strings.
Using time.Time.String():
The String() method converts a time.Time value to a string using the default format: "2006-01-02 15:04:05.999999999 -0700 MST".
t := time.Now() t_string := t.String()
Using time.Time.Format():
The Format() method allows you to specify a custom format string to customize the output of the timestamp string. For example, to format a timestamp as "2006-01-02 15:04:05", use the following format string:
t_string = t.Format("2006-01-02 15:04:05")
Example Code:
Modifying the given code to convert time.Time values to strings:
import ( "time" "fmt" ) func main() { t := time.Now() fmt.Printf("Default Format: %s\n", t.String()) fmt.Printf("Customized Format (YYYY-MM-DD): %s\n", t.Format("2006-01-02")) }
Output:
Default Format: 2023-03-08 16:12:30.6176961 +0700 WIB Customized Format (YYYY-MM-DD): 2023-03-08
By using these methods, you can successfully convert time.Time objects to strings and add them to []string arrays. This allows you to work with timestamps in your Go code and display them in a more user-friendly format.
The above is the detailed content of How to Convert Go's time.Time Objects to Strings for String Arrays?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




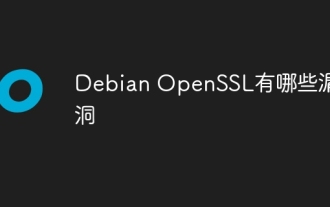
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
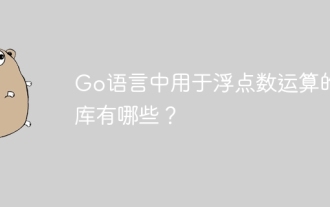
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
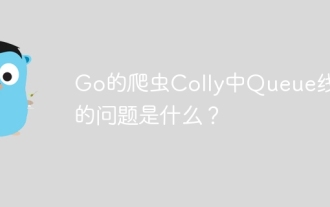
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
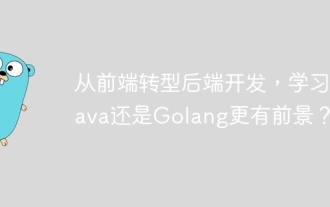
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
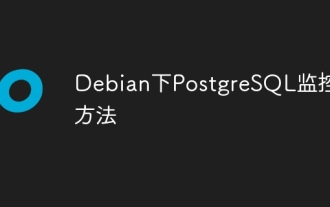
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
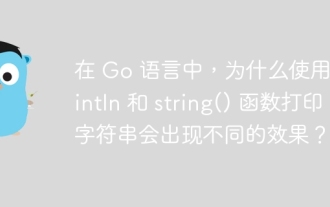
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
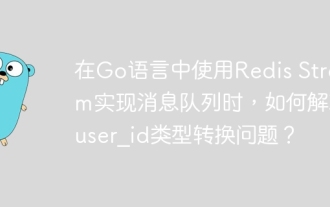
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
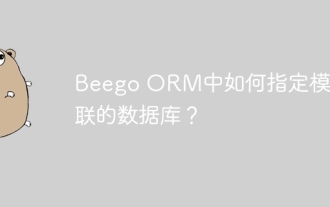
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
