


How to Safely Retrieve Map Keys in Go: Addressing Type Mismatch Issues?
How to Obtain Map Keys in Go
When retrieving keys from a map, it's crucial to ensure type compatibility. Go's strong typing requires that map keys of the same type. Unfortunately, if your Keys() function specifies a map of type map[interface{}]interface{}, but your actual map is of type map[int]interface{}, you will encounter a type mismatch error.
Go Generics and Type Compatibility
Go does not support generics, unlike languages like Java or C#. This simplifies the language and enhances performance. As a result, you cannot create generic functions that operate on maps of any key or value type.
Resolving the Type Mismatch Issue
To resolve the type mismatch, you have several options:
- Modify the Keys() function to handle map[int]interface{} explicitly, as demonstrated in this code:
func Keys(m map[int]interface{}) []int { keys := make([]int, len(m)) i := 0 for k := range m { keys[i] = k i++ } return keys }
- Alternatively, change the map to map[interface{}]interface{}, ensuring type compatibility with the Keys() function:
m2 := map[interface{}]interface{}{ 2: "string", 3: "int", }
- You can also utilize the reflect package, although it comes with performance implications.
The above is the detailed content of How to Safely Retrieve Map Keys in Go: Addressing Type Mismatch Issues?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


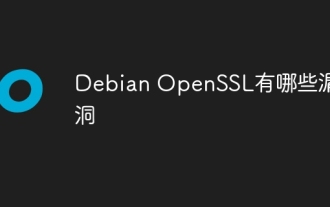
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
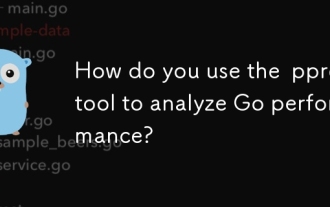
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
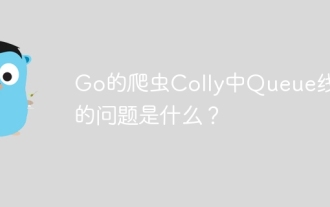
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
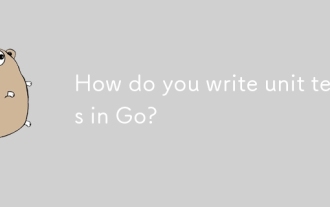
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
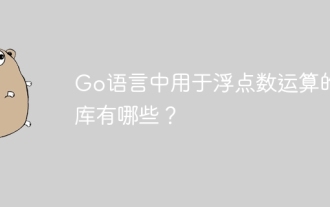
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
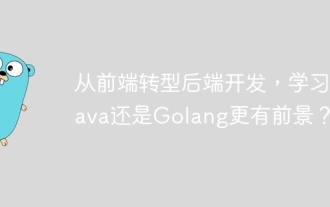
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
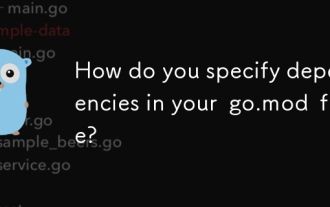
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
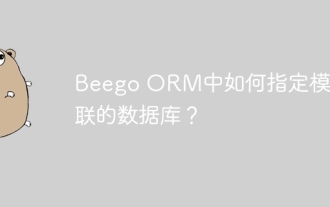
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
