


How Can I Flatten a Nested Dictionary and Compress Its Keys in Python?
Flattening Nested Dictionaries with Key Compression
When working with complex data structures in your code, it can often be useful to "flatten" them for easier manipulation. This is particularly true for nested dictionaries, where multiple levels of keys and values can add complexity to your operations. In this article, we'll explore how to flatten a nested dictionary while compressing the keys.
The Problem
Consider the following nested dictionary:
{'a': 1, 'c': {'a': 2, 'b': {'x': 5, 'y': 10}}, 'd': [1, 2, 3]}
The goal is to flatten this dictionary into something like:
{'a': 1, 'c_a': 2, 'c_b_x': 5, 'c_b_y': 10, 'd': [1, 2, 3]}
where the nested keys are compressed to create a single, flattened key.
The Solution
To flatten the nested dictionary, we can use the flatten() function from the collections.abc module. This function can be defined as follows:
def flatten(dictionary, parent_key='', separator='_'): items = [] for key, value in dictionary.items(): new_key = parent_key + separator + key if parent_key else key if isinstance(value, MutableMapping): items.extend(flatten(value, new_key, separator=separator).items()) else: items.append((new_key, value)) return dict(items)
Using the Function
To use the flatten() function, simply pass in the nested dictionary as an argument. The function will recursively traverse the dictionary, "flattening" it and compressing the keys.
>>> flatten({'a': 1, 'c': {'a': 2, 'b': {'x': 5, 'y': 10}}, 'd': [1, 2, 3]}) {'a': 1, 'c_a': 2, 'c_b_x': 5, 'd': [1, 2, 3], 'c_b_y': 10}
As you can see, the flattened dictionary has a single level of keys, with the keys being compressed to incorporate the nesting structure of the original dictionary.
The above is the detailed content of How Can I Flatten a Nested Dictionary and Compress Its Keys in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
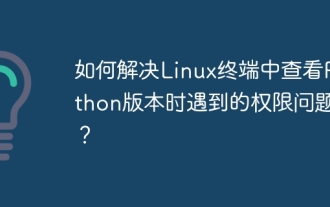
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
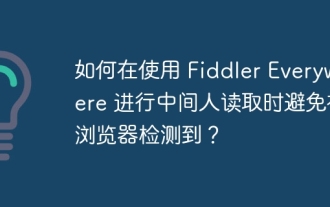
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
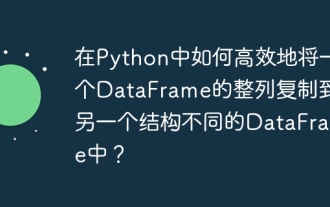
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
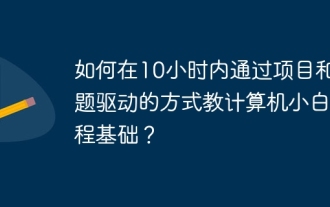
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
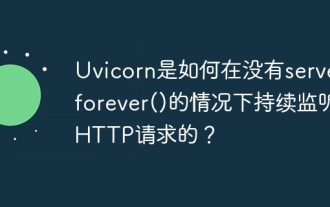
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
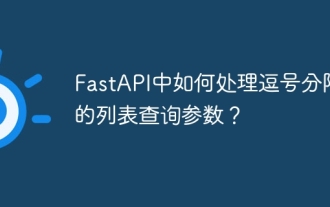
Fastapi ...
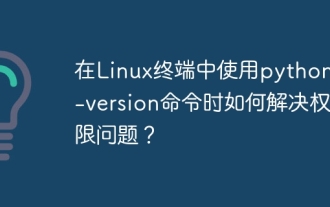
Using python in Linux terminal...
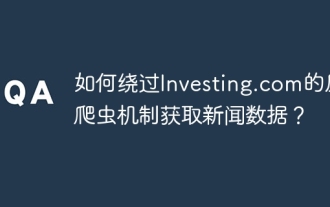
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
