Nuxt.js in action: Vue.js server-side rendering framework
Create a Nuxt.js project
First, make sure you have installed Node.js and yarn or npm. Then, create a new Nuxt.js project through the command line:
yarn create nuxt-app my-nuxt-project cd my-nuxt-project
During the creation process, you can choose whether you need options such as UI framework, preprocessor, etc., and configure them as needed.
Directory structure
Nuxt.js follows a specific directory structure, some of the key directories are as follows:
├── .nuxt/ # Automatically generated files, including compiled code and configuration ├── assets/ # Used to store uncompiled static resources, such as CSS, images, fonts ├── components/ # Custom Vue components ├── layouts/ # Application layout files, defining the general structure of the page │ └── default.vue # Default layout ├── middleware/ # Middleware files ├── pages/ # Application routes and views, each file corresponds to a route │ ├── index.vue # Default homepage │ └── [slug].vue # Dynamic routing example ├── plugins/ # Custom Vue.js plugins ├── static/ # Static resources, will be copied to the output directory as is ├── store/ # Vuex state management file │ ├── actions.js # Vuex actions │ ├── mutations.js # Vuex mutations │ ├── getters.js # Vuex getters │ └── index.js # Vuex store entry file ├── nuxt.config.js # Nuxt.js configuration file ├── package.json # Project dependencies and scripts └── yarn.lock # Or npm.lock, record dependency versions
- .nuxt/: This directory is automatically generated and contains compiled code. Generally, it does not need to be modified directly.
- assets/: Stores uncompiled static resources, such as CSS, JavaScript, and images. Nuxt.js will process these resources during the build.
- components/: Stores custom Vue components that can be reused in different parts of the application.
- layouts/: Defines the layout of the page. There can be a default layout or multiple specific layouts.
- pages/: Each file corresponds to a route, and the file name is the route name. Dynamic routes are represented by square brackets [].
- middleware/: Place custom middleware, which can execute logic before and after page rendering.
- plugins/: Custom entry file for Vue.js plugins.
- static/: Directly copied to the build output directory without any processing, often used to store robots.txt or favicon.ico, etc.
- store/: Vuex state management directory, storing actions, mutations, getters and the entry file of the entire store.
- nuxt.config.js: Nuxt.js configuration file, used to customize project settings.
- package.json: Project dependencies and script configuration.
- yarn.lock or npm.lock: Record the exact version of project dependencies to ensure dependency consistency in different environments.
Page rendering
Create an index.vue file in the pages/ directory. This is the homepage of the application:
<!-- pages/index.vue --> <template> <div> <h1>Hello from Nuxt.js SSR</h1> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { message: 'This content is server-rendered!' }; }, asyncData() { // Here you can get data on the server side // The returned data will be used as the default value of data return { message: 'Data fetched on server' }; } }; </script>
The process of Nuxt.js page rendering is divided into two main stages: server-side rendering (SSR) and client-side rendering (CSR). Here are the detailed steps of Nuxt.js page rendering:
Initialization:
The user enters the URL in the browser and sends a request to the server.
After the server receives the request, it starts processing.
Route resolution:
Nuxt.js uses the routes configuration in nuxt.config.js (if it exists) or automatically generates routes from the pages/ directory.
The corresponding page file is identified, such as pages/index.vue or pages/about.vue.
Data prefetching:
Nuxt.js looks for asyncData or fetch methods in the page component (if they exist).
These methods will run on the server side to get data from APIs or other data sources.
After the data is obtained, it will be serialized and injected into the page template.
Template rendering:
Nuxt.js uses Vue.js's rendering engine to convert components and pre-fetched data into an HTML string.
The HTML string contains all the initial data required by the client, inlined in the

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


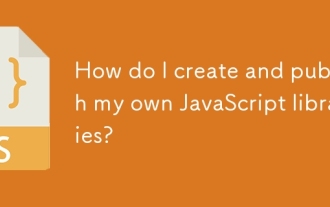
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
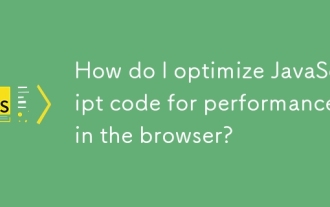
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
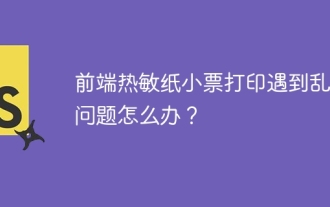
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
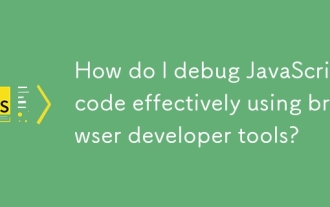
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
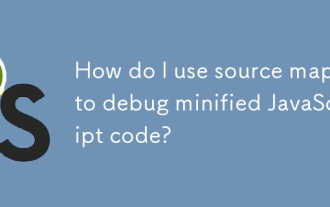
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
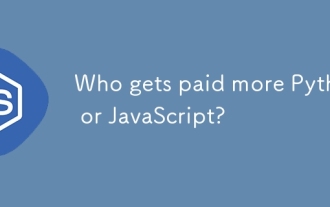
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
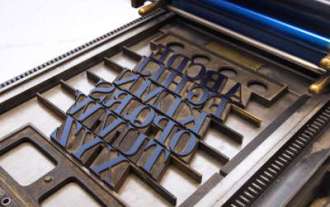
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
