


How to Efficiently Create ASP.NET Controls within Dynamically Created ASP.NET Controls?
How to Dynamically Create ASP.NET Controls within Dynamically Created ASP.NET Controls
The task of generating HTML, ASP textboxes, and buttons within dynamically created controls can be a complex one. Fortunately, there are several approaches you can take to achieve this.
Approach 1: Manually Generating HTML and Controls
In your example, you're manually generating HTML within code, then dynamically creating textboxes and buttons using the controls framework. While this method works, it can become tedious and error-prone as the number of generated controls increases.
Approach 2: Using a ListView or DataGrid
For generating a series of similar controls, such as a list of hazards or areas, using a ListView or DataGrid is a recommended approach. These controls provide built-in support for data binding, allowing you to easily render controls based on a data source.
Approach 3: Using Client-Side Scripting
If you're comfortable with client-side scripting, you can use JavaScript or jQuery to dynamically create and manipulate elements on the fly. This approach eliminates the need for server-side code and allows for more interactive functionality.
Code Example: Using a ListView
Here's an example using a ListView to generate a list of hazards within dynamically created areas:
private void CreateArea(int areaCount) { // ... existing code // Create a ListView for hazards ListView hazardList = new ListView(); hazardList.ID = "lvHazards" + areaCount; hazardList.DataSource = new string[] { "Hazard1", "Hazard2", "Hazard3" }; hazardList.DataBind += HazardList_DataBind; phHazard.Controls.Add(hazardList); } protected void HazardList_DataBind(object sender, ListViewDataBindEventArgs e) { ListView listView = (ListView)sender; foreach (string hazard in e.DataItem as string[]) { // Create a new DropDownList for the hazard DropDownList ddHazard = new DropDownList(); ddHazard.ID = "ddHazard" + hazard; ddHazard.Items.Add(new ListItem("Hazard1", "Hazard1")); // Create a new TextBox for the hazard description TextBox txtHazardDesc = new TextBox(); txtHazardDesc.ID = "txtHazardDesc" + hazard; // Add the controls to the current ListView item listView.Controls.Add(ddHazard); listView.Controls.Add(new LiteralControl(" ")); listView.Controls.Add(txtHazardDesc); listView.Controls.Add(new LiteralControl("<br />")); } }
This example shows how to create a ListView to display a list of hazards within each dynamically created area. The ListView's data binding is handled in the HazardList_DataBind event handler, where controls are dynamically created and added to the appropriate ListView item.
Remember that dynamically created controls will need to be recreated on every page postback to maintain their state. Consider using ViewState or a caching mechanism to store data associated with dynamic controls to prevent them from being lost.
The above is the detailed content of How to Efficiently Create ASP.NET Controls within Dynamically Created ASP.NET Controls?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
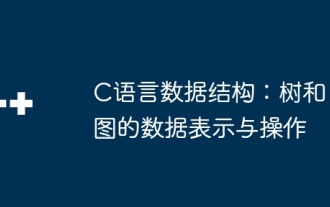
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
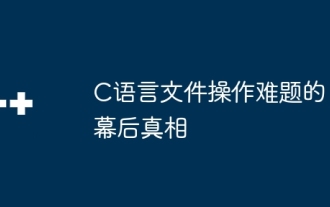
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
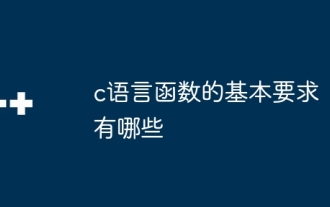
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
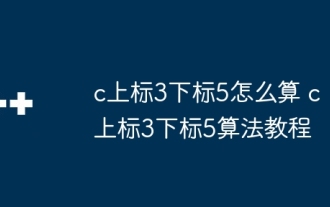
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
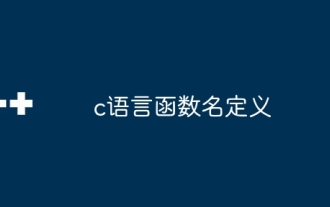
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
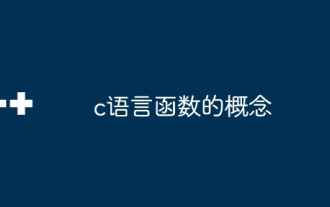
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
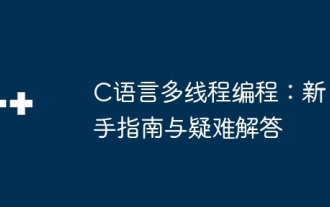
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
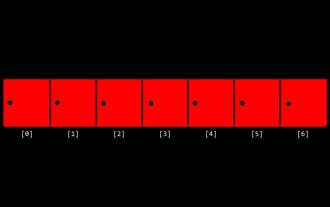
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
