How to List a Directory's Contents in Go Without Recursion?
Listing Directory Contents in Go Without Recursion
In Go, listing files and folders within a specified directory can be accomplished by leveraging the ReadDir function from the os package. Unlike filepath.Walk, which traverses directories recursively, ReadDir limits its scope to the designated directory.
The ReadDir function returns a slice of os.DirEntry objects, which provide information about each directory entry, including filename and file type. To list the contents of a directory without delving into subdirectories, follow these steps:
package main import ( "fmt" "os" "log" ) func main() { entries, err := os.ReadDir("./") if err != nil { log.Fatal(err) } for _, e := range entries { fmt.Println(e.Name()) } }
In this example, the ReadDir function reads the current directory's contents and stores them in the entries slice. Each os.DirEntry in the slice contains the entry's name, which is then printed to the console.
This approach provides a straightforward method to list the files and folders within a specific directory without exploring subdirectories.
The above is the detailed content of How to List a Directory's Contents in Go Without Recursion?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
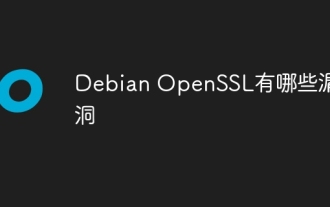
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
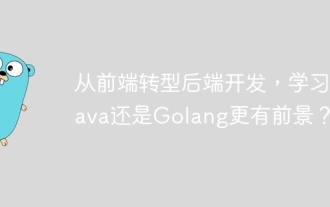
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
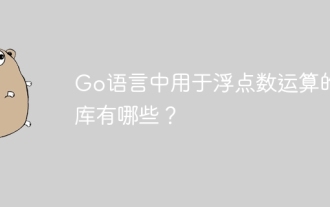
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
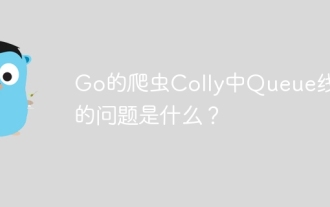
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
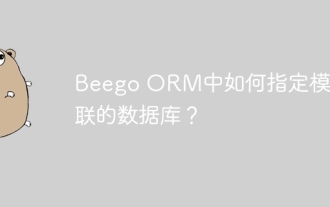
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
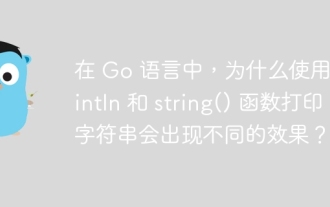
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
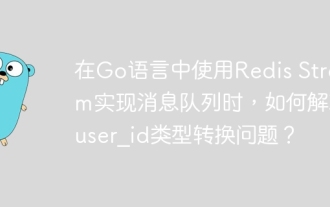
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
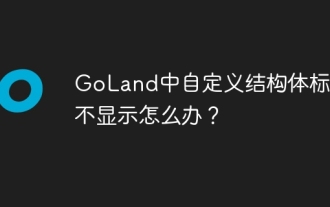
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
