How to Best Handle Nullable Time Values in Go Structs?
Handling Nullable Time Values with Structs in Go
When working with data structures that may contain nullable time values, it's important to ensure proper handling of these values. Consider the following struct:
1 2 3 4 5 6 7 |
|
Here, the RemindedAt field is declared as a pointer to a time.Time, as it's possible for it to be null. However, this distinction requires the code to handle the difference between CreatedAt and RemindedAt.
To address this, Go provides several approaches to handle nullable time values elegantly:
Using pq.NullTime
The pq package from the PostgreSQL Go driver offers the pq.NullTime type. It consists of a time.Time value and a Valid boolean flag indicating whether the time is valid (not NULL).
1 2 3 4 5 6 7 8 9 |
|
In this case, RemindedAt is a pq.NullTime value, and the code can check its Valid flag to determine if the time is set.
Using sql.NullTime (Go 1.13 and above)
Starting with Go 1.13, the standard library introduces the sql.NullTime type, which serves a similar purpose to pq.NullTime.
1 2 3 4 5 6 7 8 9 |
|
Both pq.NullTime and sql.NullTime implement the necessary interfaces to support database scanning and parameter binding, making them convenient to use with database operations.
The above is the detailed content of How to Best Handle Nullable Time Values in Go Structs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
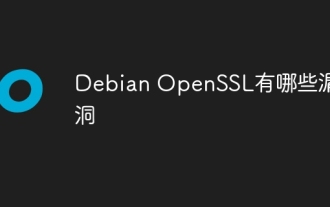
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
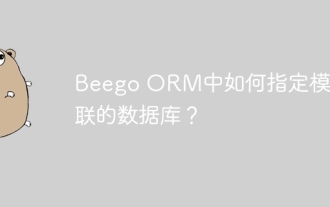
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
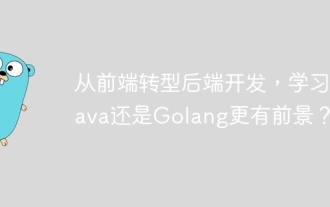
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
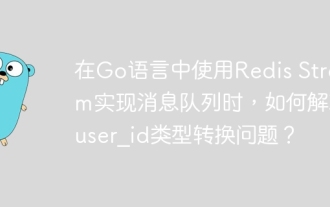
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
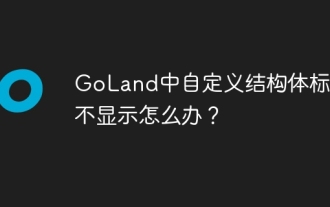
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
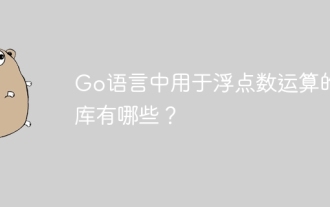
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
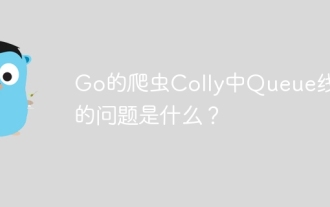
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
