What is SQL Injection? and how to prevent
SQL Injection
It is a security attack that is common in database systems that use SQL (Structured Query Language) as the language for database management. This attack occurs when an attacker injects malicious SQL code into user input fields, such as form fields or URLs, to improperly access or modify data in a database.
SQL Injection ranks #3 in the 2021 OWASP Top 10, a list of the most common and important security vulnerabilities for web applications by the Open Web Application Security Project (OWASP). This is updated every time. over several years to reflect changing cyber threats
SQL injection attacks are ranked as the #3 threat, demonstrating the importance and prevalence of this issue in terms of web application security. Properly dealing with injection attacks is essential to securing information systems and preventing unwanted access
How to attack SQL Injection
SQL Injection attacks can be done in many different ways, but there are two most common ones:
- Inserting values directly into the input field
- Editing URL to add SQL statement
Example of a SQL Injection attack
Suppose there is a web application that allows users to log in, with PHP code
<?php $username = $_POST['username']; $password = $_POST['password']; $query = "SELECT * FROM users WHERE username='$username' AND password='$password'"; $result = mysqli_query($conn, $query); if (mysqli_num_rows($result) > 0) { echo "Login successful!"; } else { echo "Invalid username or password."; } ?>
This code is vulnerable because inserting user values directly into SQL statements can cause SQL injection
SQL Injection Attacks
Hackers can insert malicious values into data fields like this
- Username field ' OR '1'='1
- password field ' OR '1'='1
This makes the SQL statement look like this
SELECT * FROM users WHERE username='' OR '1'='1' AND password='' OR '1'='1'
Because '1'='1' is a condition that is always true. This command returns information for every user in the database. This allows hackers to log in without using a real password
How to prevent SQL Injection
There are many ways to prevent SQL Injection such as
- Using Prepared Statements
- Using Stored Procedures
- Filtering and validating input data. ## Example of using Prepared Statements in PHP
<?php $stmt = $conn->prepare("SELECT * FROM users WHERE username=? AND password=?"); $stmt->bind_param("ss", $username, $password); $username = $_POST['username']; $password = $_POST['password']; $stmt->execute(); $result = $stmt->get_result(); if ($result->num_rows > 0) { echo "Login successful!"; } else { echo "Invalid username or password."; } ?>
In this example, the SQL statement is prepared in advance, and the $username and $password values are inserted into the statement later. This makes it impossible to inject malicious SQL code
summarize
SQL Injection is a serious threat. But this can be prevented by writing secure code, such as using Prepared Statements and validating input. Good protection doesn't just help keep your data safe. But it also helps maintain the reliability of the system.
The above is the detailed content of What is SQL Injection? and how to prevent. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










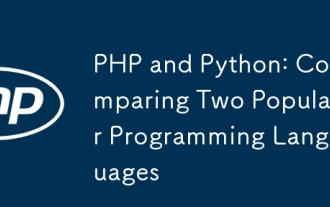
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
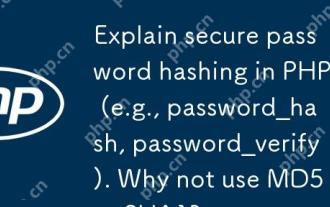
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
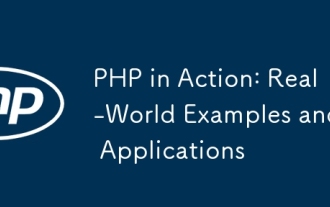
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
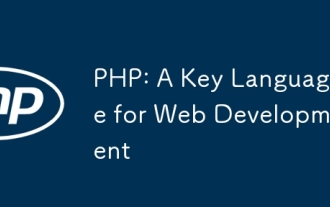
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
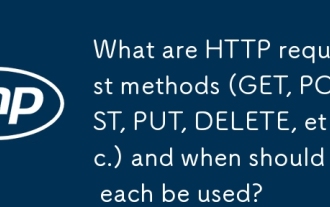
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
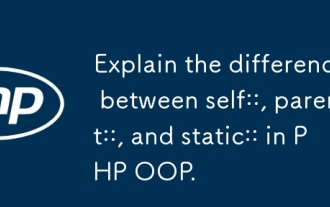
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
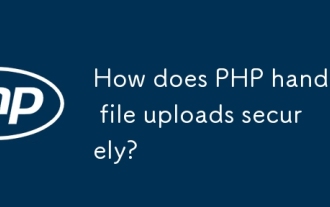
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
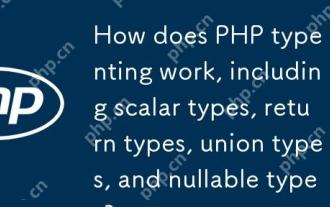
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
