How Can I Handle Default Value Returns for Generic Types in Go?
Implementing Default Value Returns for Generic Types in Go
In Go, the lack of a null value in the language presents a challenge when returning an empty or default value for generic types.
Limitations of Nil Returns
Consider the following generic list implementation:
type mylist[T any] struct { first *node[T] } type node[T any] struct { data T next *next[T] }
In this example, we might define methods for popping and retrieving the first element from the list:
func (list *mylist[T]) pop() T { if list.first != nil { data := list.first.data list.first = list.first.next return data } return nil } func (list *mylist[T]) getfirst() T { if list.first != nil { return list.first.data } return nil }
However, when compiling this code, the compiler will raise an error: "cannot use nil as T value in return statement." This occurs because Go cannot implicitly assign nil to a generic type variable T.
Solution: Returning the Zero Value
Instead of returning nil, we can return the zero value for the specific type T used in the generic list. The zero value is:
- Nil for pointers
- Empty string for strings
- 0 for integers and floating-point numbers
To achieve this, we can declare a variable of type T and return it:
func getZero[T any]() T { var result T return result }
This function can now be used to return the zero value for any generic type. For instance, we can test it for different types:
i := getZero[int]() fmt.Printf("%T %v\n", i, i) s := getZero[string]() fmt.Printf("%T %q\n", s, s) p := getZero[image.Point]() fmt.Printf("%T %v\n", p, p) f := getZero[*float64]() fmt.Printf("%T %v\n", f, f)
Output:
int 0 string "" image.Point (0,0) *float64 <nil>
This solution enables you to return a proper default or empty value for any generic type in Go.
The above is the detailed content of How Can I Handle Default Value Returns for Generic Types in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










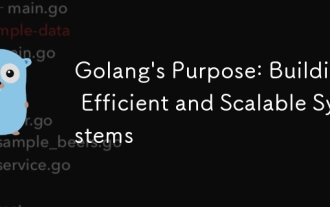
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
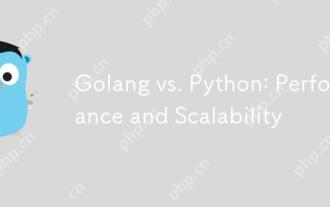
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
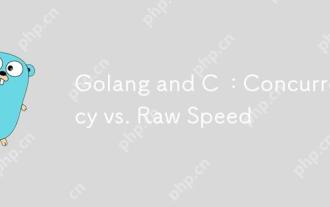
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
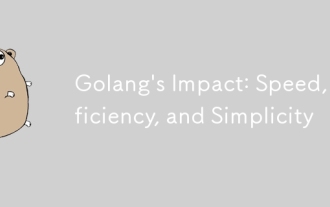
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
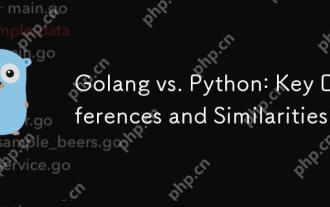
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
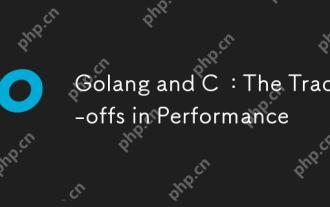
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
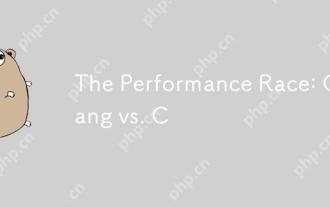
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
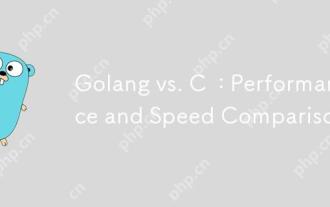
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
