


How to Retrieve a Pixel Array from a Go `image.Image` for OpenGL ES 2.0?
Pixel Array Retrieval from Go Image.Image
In Go, the image.Image interface represents an image containing pixel data. To obtain a pixel array from an image.Image for use in OpenGL ES 2.0 via the texImage2D method, you can use the following steps:
- Define a custom Pixel struct to represent individual pixels:
type Pixel struct { R int G int B int A int }
- Create a function, rgbaToPixel, to convert RGBA values to a Pixel struct:
func rgbaToPixel(r uint32, g uint32, b uint32, a uint32) Pixel { return Pixel{int(r / 257), int(g / 257), int(b / 257), int(a / 257)} }
- Write a function, getPixels, to extract the pixel array from the image.Image:
func getPixels(img image.Image) ([][]Pixel, error) { bounds := img.Bounds() width, height := bounds.Max.X, bounds.Max.Y var pixels [][]Pixel for y := 0; y < height; y++ { var row []Pixel for x := 0; x < width; x++ { row = append(row, rgbaToPixel(img.At(x, y).RGBA())) } pixels = append(pixels, row) } return pixels, nil }
- Load an image and call getPixels to obtain the pixel array:
file, err := os.Open("./image.png") if err != nil { fmt.Println("Error: File could not be opened") os.Exit(1) } defer file.Close() pixels, err := getPixels(file) if err != nil { fmt.Println("Error: Image could not be decoded") os.Exit(1) }
- Use pixels to pass to the texImage2D method as needed.
The above is the detailed content of How to Retrieve a Pixel Array from a Go `image.Image` for OpenGL ES 2.0?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










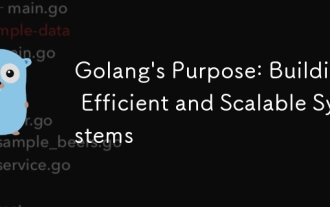
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
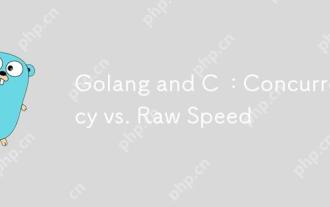
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
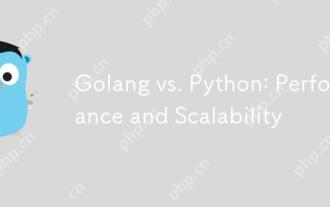
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
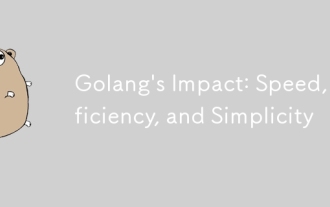
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
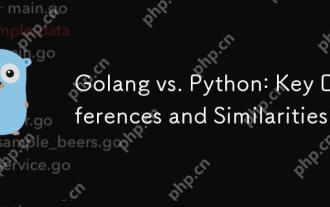
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
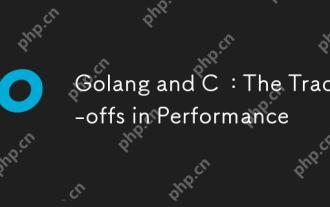
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
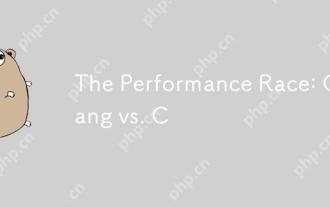
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
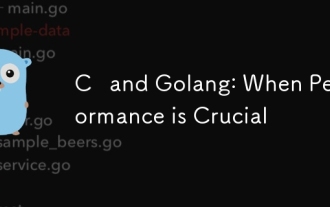
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
