


How Can I Await Any Event on Any Type Using a General-Purpose FromEvent Method?
General Purpose FromEvent Method
The original FromEvent method requires creating separate methods for each event and class you want to await on. To eliminate boilerplate code, developers sought a more general-purpose solution.
One approach involves using reflection to retrieve the event and its delegate type. However, accessing the delegate's parameters and modifying TaskCompletionSource posed challenges.
A Custom Solution
Here's a custom solution that utilizes IL emission and event handlers:
public static class ExtensionMethods { public static Task<object[]> FromEvent<T>(this T obj, string eventName) { // Create a TaskCompletionSourceHolder to manage the TaskCompletionSource and event handler var tcsh = new TaskCompletionSourceHolder(); // Get the event and its delegate type EventInfo eventInfo = obj.GetType().GetEvent(eventName); Type eventDelegateType = eventInfo.EventHandlerType; // Create a dynamic method to handle the event DynamicMethod handler = CreateEventHandler(eventDelegateType); // Add the event handler to the target object eventInfo.AddEventHandler(obj, handler.CreateDelegate(eventDelegateType, tcsh)); // Return the Task from the TaskCompletionSourceHolder return tcsh.Task; } private static DynamicMethod CreateEventHandler(Type eventDelegateType) { // Get the parameter types of the event delegate var parameterTypes = GetDelegateParameterTypes(eventDelegateType); // Insert the TaskCompletionSourceHolder as the first parameter parameterTypes.Insert(0, typeof(TaskCompletionSourceHolder)); // Create a new dynamic method and IL generator DynamicMethod handler = new DynamicMethod("EventHandler", typeof(void), parameterTypes, true); ILGenerator ilgen = handler.GetILGenerator(); // Load the TaskCompletionSourceHolder and create an array to store the arguments ilgen.Emit(OpCodes.Ldarg_0); ilgen.Emit(OpCodes.Ldc_I4, parameterTypes.Count - 1); ilgen.Emit(OpCodes.Newarr, typeof(object)); // Store each argument in the array for (int i = 1; i < parameterTypes.Count; i++) { ilgen.Emit(OpCodes.Ldloc_0); ilgen.Emit(OpCodes.Ldc_I4, i - 1); ilgen.Emit(OpCodes.Ldarg, i); ilgen.Emit(OpCodes.Stelem_Ref); } // Call the SetResult method on the TaskCompletionSourceHolder ilgen.Emit(OpCodes.Call, typeof(TaskCompletionSourceHolder).GetMethod("SetResult")); // Return from the method ilgen.Emit(OpCodes.Ret); return handler; } private static List<Type> GetDelegateParameterTypes(Type delegateType) { var parameters = delegateType.GetMethod("Invoke").GetParameters(); var parameterTypes = parameters.Select(p => p.ParameterType).ToList(); return parameterTypes; } }
With this method, you can now await any event on any type:
await new MyClass().FromEvent("MyEvent");
Benefits of this Solution
- Supports any event type with any number or types of parameters.
- Does not require casting the Task to a specific return type.
- Eliminates the need for creating multiple FromEvent methods for different types and events.
The above is the detailed content of How Can I Await Any Event on Any Type Using a General-Purpose FromEvent Method?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










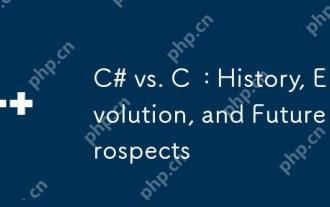
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
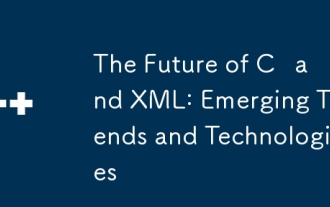
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
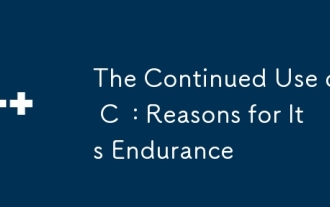
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
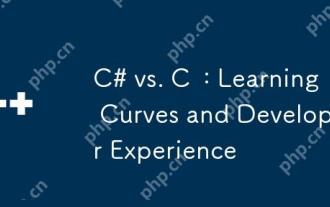
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
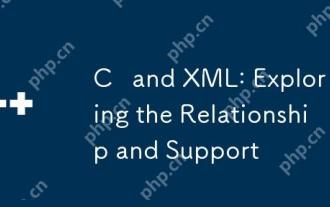
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
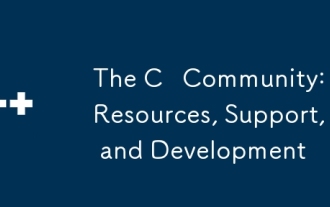
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
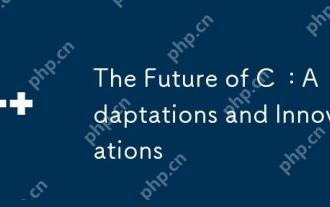
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
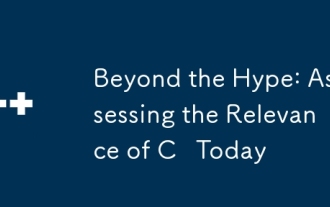
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
