


Why Does Python's `input()` Function Cause 'NameError' and How Can I Avoid It?
Input() Error: Handling Name Errors in Python
When attempting to run a script that utilizes the input function, you may encounter the error "NameError: name '...' is not defined." This occurs when the input function's attempt to evaluate the user's input as a Python expression fails due to the absence of a valid name or variable.
Understanding Input() in Python 2.7
In Python 2.7, the input function executes a unique behavior. It interprets the user's input as a Python expression. If the script expects a string, the input should not be enclosed in quotes. However, when a string is expected but is wrapped in quotes, the error occurs due to the presence of the additional quotes.
For example:
input_variable = input("Enter your name: ") # Expecting a string without quotes print("Your name is " + input_variable) # Error: NameError
To rectify this in Python 2.7, utilize the raw_input function instead. This function simply reads the user's input without interpreting it as a Python expression.
input_variable = raw_input("Enter your name: ") # Expecting a string with quotes print("Your name is " + input_variable) # No error
Input() in Python 3.x
In Python 3.x, the raw_input function has been renamed to input. Therefore, it behaves in a similar manner to raw_input in Python 2.7: it reads the user's input as a string without interpretation.
Security Implications of Input() in Python 2.7
Since input in Python 2.7 evaluates user input, it poses security concerns. If a malicious user inputs a command that is not properly sanitized or validated, it could potentially execute malicious code. For example, if a script has imported the os module and a user enters the following as input:
os.remove("/etc/hosts")
This could result in the deletion of the /etc/hosts file, highlighting the importance of using raw_input instead for reading sensitive user input.
The above is the detailed content of Why Does Python's `input()` Function Cause 'NameError' and How Can I Avoid It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










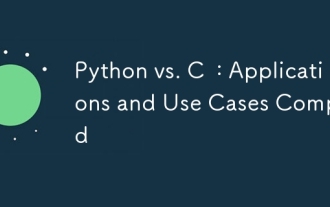
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
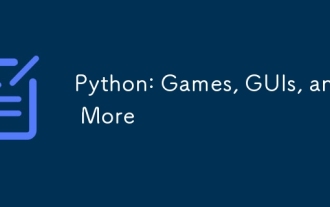
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
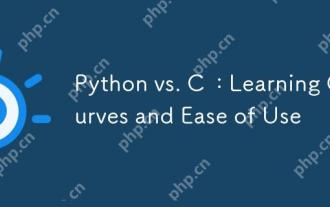
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
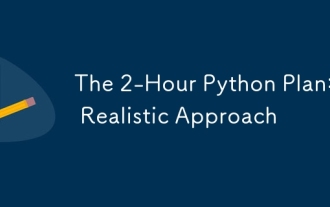
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
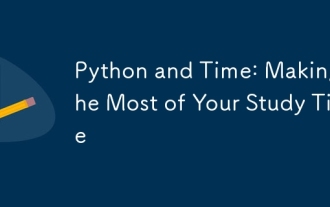
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
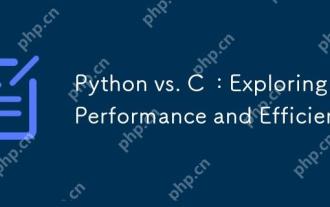
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
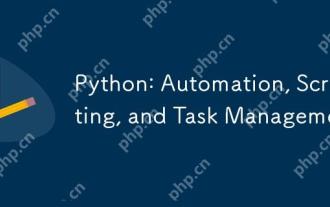
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
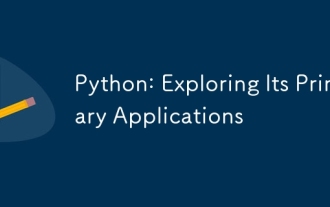
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
