


How to Sort an Array of Associative Arrays by a Column Value in PHP?
Sorting an Array of Associative Arrays by Column Value
Sorting data is a fundamental task in programming. When it comes to associative arrays, PHP provides several built-in functions to facilitate this process.
Consider the following array of associative arrays:
$inventory = array( array("type" => "fruit", "price" => 3.50), array("type" => "milk", "price" => 2.90), array("type" => "pork", "price" => 5.43) );
The task is to sort the elements of $inventory by the "price" column in descending order, resulting in:
$inventory = array( array("type" => "pork", "price" => 5.43), array("type" => "fruit", "price" => 3.50), array("type" => "milk", "price" => 2.90) );
Solution using array_multisort()
The array_multisort() function allows sorting an array (or multiple arrays) by multiple columns. To sort by "price" in descending order, the following code can be utilized:
$price = array(); foreach ($inventory as $key => $row) { $price[$key] = $row['price']; } array_multisort($price, SORT_DESC, $inventory);
Alternative Solution with array_column() (PHP 5.5.0 )
For PHP versions 5.5.0 and above, the array_column() function can be used to simplify the above code:
$price = array_column($inventory, 'price'); array_multisort($price, SORT_DESC, $inventory);
Usage
The sorted $inventory array can now be used as needed. For example, the following code would print the sorted array:
foreach ($inventory as $item) { echo $item['type'] . ": $" . $item['price'] . PHP_EOL; }
Output:
pork: .43 fruit: .50 milk: .90
The above is the detailed content of How to Sort an Array of Associative Arrays by a Column Value in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




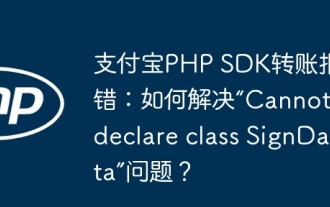
Alipay PHP...
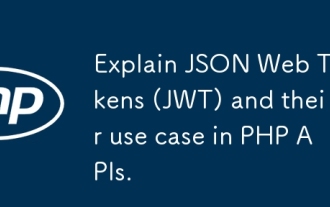
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
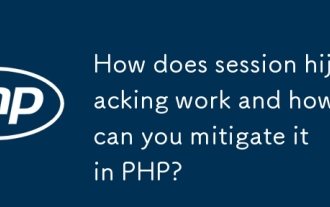
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
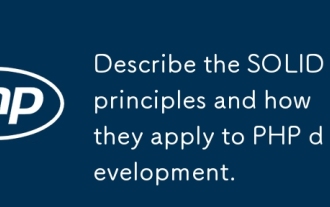
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
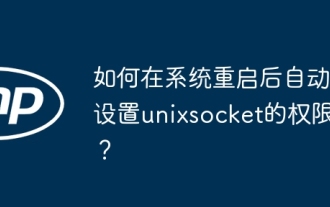
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
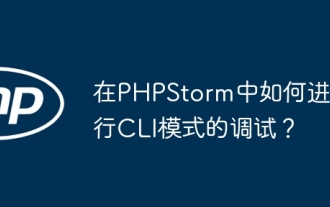
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
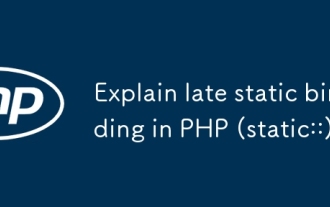
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
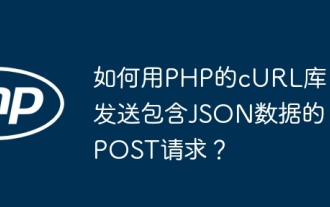
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
