


How to Display MySQL Database Table Data as an HTML Table on a Webpage?
Show Values from MySQL Database Table in HTML Table on a Webpage
Querying the Database
To retrieve the values from a MySQL database table, you'll first need to connect to the database and execute a query. Consider the following PHP code:
$con = mysqli_connect("localhost", "database_user", "database_password", "database_name"); $result = mysqli_query($con, "SELECT * FROM tickets");
Here, we connect to the database using mysqli_connect and then execute the SELECT * FROM tickets query to fetch all the rows from the tickets table.
Displaying the Results in HTML Table
Once the results are retrieved, you can use HTML to create a table and display the values.
echo "<table border='1'>"; echo "<tr><th>Submission ID</th><th>Form ID</th><th>IP</th><th>Name</th><th>E-mail</th><th>Message</th></tr>"; while ($row = mysqli_fetch_array($result)) { echo "<tr><td>{$row['submission_id']}</td><td>{$row['formID']}</td><td>{$row['IP']}</td><td>{$row['name']}</td><td>{$row['email']}</td><td>{$row['message']}</td></tr>"; } echo "</table>";
In this code, we start by creating a table with headers. Then, we use a while loop to iterate through the result and create a new row for each row in the table. The values from each row are obtained using the $row['column_name'] syntax.
Complete Code Example
Combining the query and display code, we get the following complete code:
This code will retrieve the values from the tickets table and display them in an HTML table on a webpage.
The above is the detailed content of How to Display MySQL Database Table Data as an HTML Table on a Webpage?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
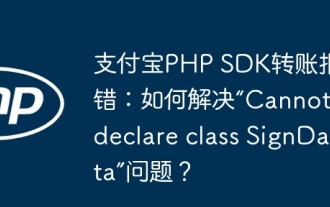
Alipay PHP...
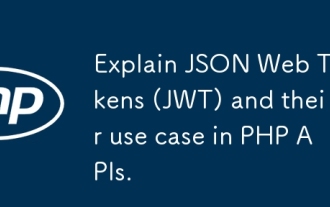
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
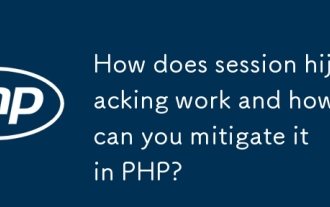
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
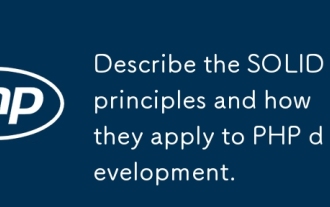
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
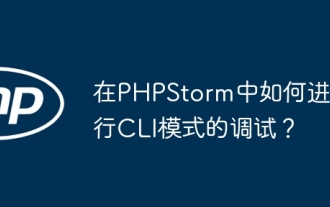
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
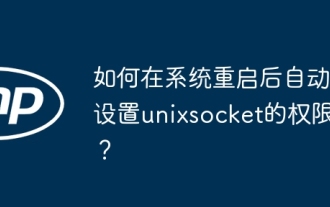
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
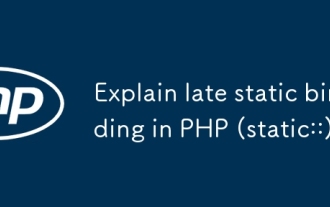
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
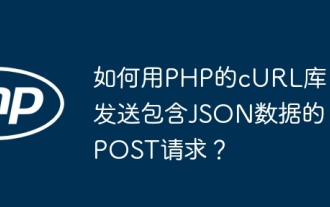
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
