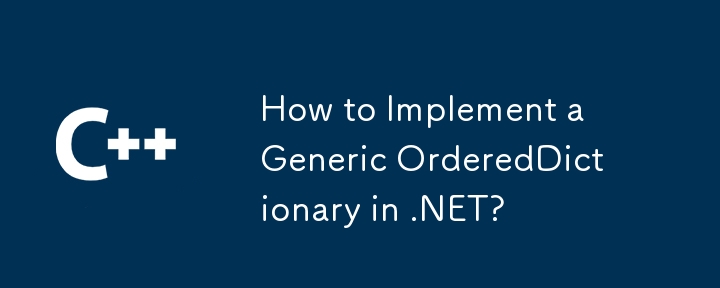
Implementing OrderedDictionary
Implementing a generic OrderedDictionary is not a complex task, but it can be time-consuming and is considered an oversight on Microsoft's part. There are various methods for implementing it, but one approach involves using a KeyedCollection for internal storage and a Key and List hybrid for sorting in a similar way to List.
Class Structure
The interface for the generic OrderedDictionary (IOrderedDictionary) includes both generic and non-generic (System.Collections.Specialized.IOrderedDictionary) interfaces. The implementation class (OrderedDictionary) inherits from the KeyedCollectionBase class with a private field of type KeyedCollection2> to store the key-value pairs internally.
Overridden Methods
The OrderedDictionary class overrides various methods from both the IDictionary and IOrderedDictionary interfaces:
- Methods related to key-value management (Add, Clear, ContainsKey, Remove)
- Indexer properties to access values by key or index (this[TKey key], this[int index])
- Methods for manipulating the order of elements (Insert, IndexOf, RemoveAt)
- Methods to retrieve or set key-value pairs by index (GetValue, SetValue, GetItem, SetItem)
- Enumerator and property implementations for iterating through the collection (GetEnumerator, Count, Keys, Values)
Sorting Capabilities
The OrderedDictionary class includes methods to sort keys or values in different ways, using built-in or custom comparers:
- SortKeys(): Sorts keys by their natural order.
- SortKeys(IComparer comparer): Sorts keys using the specified comparer.
- SortKeys(Comparison comparison): Sorts keys using the specified comparison delegate.
- SortValues(): Sorts values by their natural order.
- SortValues(IComparer comparer): Sorts values using the specified comparer.
- SortValues(Comparison comparison): Sorts values using the specified comparison delegate.
Additional Features
The OrderedDictionary class also supports features such as:
- Access to the internal KeyedCollection through the _keyedCollection property.
- Sorting algorithms inherited from the KeyedCollection2 class (SortByKeys, Sort, SortByKeys).
- Helper methods to create DictionaryEnumerators for use with IOrderedDictionary.
Example Usage
The provided code example demonstrates how to create, manipulate, and sort an OrderedDictionary instance:
- Creating an OrderedDictionary with key-value pairs.
- Retrieving and setting values using indexers and GetValue/SetValue methods.
- Using the SortKeys and SortValues methods to sort the dictionary by keys or values.
- Enumerating through the collection to access each key-value pair.
Conclusion
While .NET does not natively provide a generic implementation of OrderedDictionary, the code sample provided offers a robust alternative that can be tailored to specific needs. This implementation fills the gap in .NET's collection libraries, enabling efficient and organized management of data with both key-based and index-based access.
The above is the detailed content of How to Implement a Generic OrderedDictionary in .NET?. For more information, please follow other related articles on the PHP Chinese website!