


How to Extract Substrings in PHP Using Different Delimiter Types?
Determining Substrings in PHP
In PHP, obtaining substrings within specified delimiters can be achieved using various methods. For instance, if the demarcation delimiters are identical at both ends (e.g., [foo] and [/foo]), the following approach will suffice:
function get_string_between($string, $start, $end) { $string = ' ' . $string; $ini = strpos($string, $start); if ($ini == 0) { return ''; } $ini += strlen($start); $len = strpos($string, $end, $ini) - $ini; return substr($string, $ini, $len); }
To illustrate, consider the following example:
$fullstring = 'this is my [tag]dog[/tag]'; $parsed = get_string_between($fullstring, '[tag]', '[/tag]'); echo $parsed; // (result = dog)
Additionally, if the delimiters are unique (e.g., [foo] and [/foo2]), a modified function can be employed:
function get_inner_substring($str, $delim) { $results = array(); $delim_len = strlen($delim); $pos = strpos($str, $delim); while ($pos !== false) { $pos_end = strpos($str, $delim, $pos + $delim_len); if ($pos_end !== false) { $results[] = substr($str, $pos + $delim_len, $pos_end - $pos - $delim_len); $pos = strpos($str, $delim, $pos_end + $delim_len); } else { break; } } return $results; }
Using this function, multiple substrings can be extracted from a string separated by different delimiters:
$string = " foo I like php foo, but foo I also like asp foo, foo I feel hero foo"; $arr = get_inner_substring($string, "foo"); print_r($arr); // (result = ['I like php', 'I also like asp', 'I feel hero'])
The above is the detailed content of How to Extract Substrings in PHP Using Different Delimiter Types?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










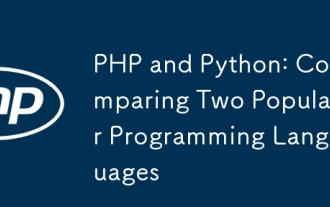
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
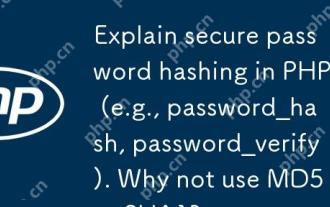
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
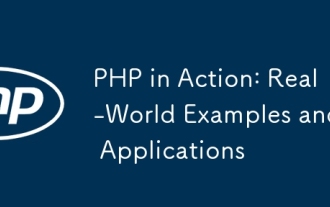
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
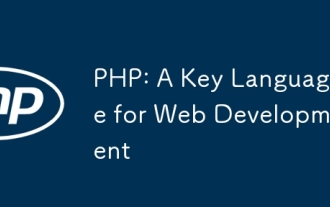
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
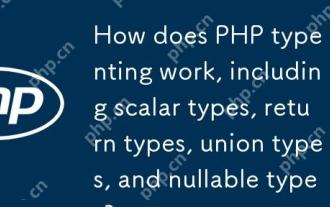
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
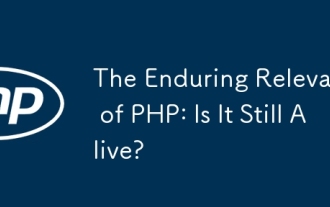
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
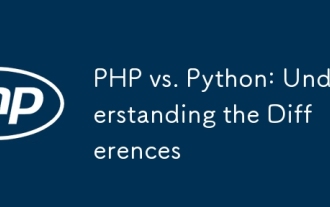
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
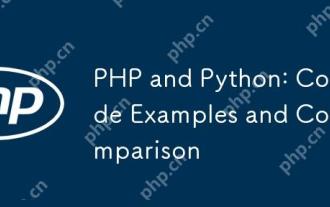
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
