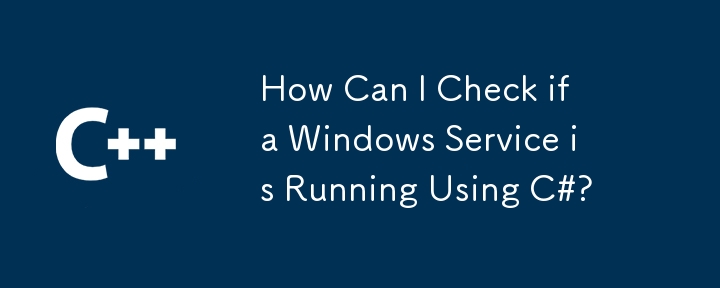
Verifying the Status of a Windows Service in C#
In an application that communicates with a Windows Service, it's crucial to determine if the service is running before initiating communication. Here's how you can accomplish this in C#:
-
Add the System.ServiceProcess Namespace:
Include using System.ServiceProcess; in your project to access the necessary ServiceController object.
-
Create a ServiceController Instance:
Instantiate a ServiceController object specifying the name of the service you want to check (e.g., ServiceController sc = new ServiceController(SERVICENAME);).
-
Retrieve the Service Status:
The Status property of the ServiceController provides the current status of the service. Access it to determine if the service is running.
-
Interpret the Status Value:
The Status property can return different values, each corresponding to a specific state of the service:
- ServiceControllerStatus.Running
- ServiceControllerStatus.Stopped
- ServiceControllerStatus.Paused
- ServiceControllerStatus.StopPending
- ServiceControllerStatus.StartPending
- ServiceControllerStatus.StatusChanging
-
Refresh the Status (Optional):
If you need to retrieve the status again, call sc.Refresh() before accessing the Status property again.
Tips:
- The ServiceController also provides a WaitforStatus() method that allows waiting for a specific status and timeout.
- You can customize the error handling for different status values if needed.
- Refer to the Microsoft documentation for more detailed information on the ServiceController object.
The above is the detailed content of How Can I Check if a Windows Service is Running Using C#?. For more information, please follow other related articles on the PHP Chinese website!