How to Partially Unmarshal JSON into a Go Map?
Unmarshalling Partial JSON into a Map in Go
Partially unmarshalling JSON data into a map can be useful when the JSON is structured in a specific way, with a key-value structure where the key identifies the type of value. This approach enables efficient processing and type-specific handling of the data.
Consider the following JSON example:
{ "sendMsg":{"user":"ANisus","msg":"Trying to send a message"}, "say":"Hello" }
To parse this JSON using the "encoding/json" package, you can unmarshal it into a map of strings to JSON "RawMessage" objects:
var objmap map[string]json.RawMessage err := json.Unmarshal(data, &objmap)
// Accessing the "sendMsg" value: var s sendMsg err = json.Unmarshal(objmap["sendMsg"], &s) // Accessing the "say" value: var str string err = json.Unmarshal(objmap["say"], &str)
To unmarshal into specific data types, you need to export the struct fields in your sendMsg struct:
type sendMsg struct { User string Msg string }
This approach provides flexibility in handling JSON data with varying structures and allows for type-safe unmarshalling based on the key in the JSON object.
The above is the detailed content of How to Partially Unmarshal JSON into a Go Map?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
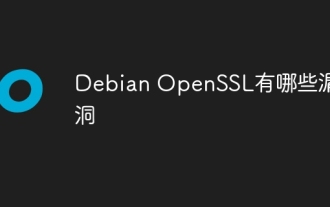
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
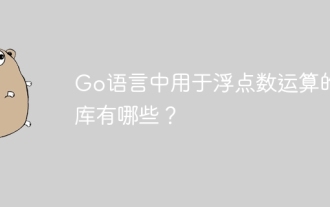
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
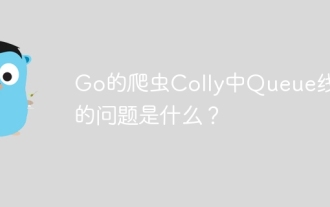
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
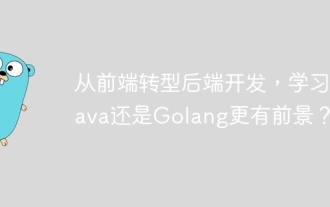
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
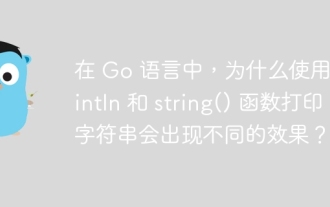
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
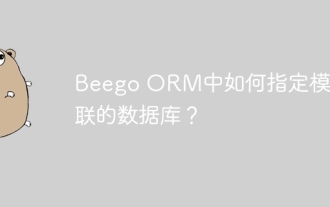
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
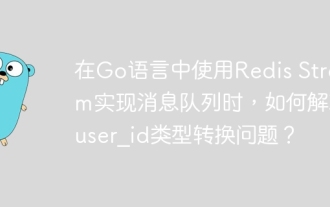
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
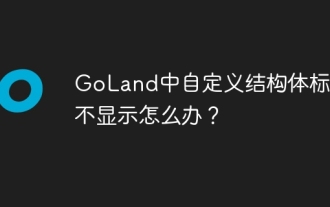
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
