


How Can I Efficiently Serialize Go Structs to Disk with Minimal File Size?
Efficient Go Serialization of Struct to Disk: Achieving Minimal Bloat
Despite the bloated output produced by gob serialization, a deeper analysis reveals that subsequent entries of the same type incur only a 12-byte overhead. This overhead represents the minimum size required to encode two strings of length 4 bytes (including length prefixes).
To reduce the overall file size, consider the following strategies:
- Use Multiple Encoder Instances: Amortizing the compilation cost of the custom codec across multiple encoders can significantly reduce the overhead for the first entry.
- Compress the Output: Using compression libraries like compress/flate or bzip2 can further reduce the file size, with bzip2 achieving the highest efficiency in the provided test (2.04 bytes/Entry).
Code Demonstration:
The following Go code demonstrates the various approaches discussed:
package main import ( "bytes" "compress/bzip2" "compress/flate" "compress/gzip" "compress/zlib" "encoding/gob" "fmt" "io" ) type Entry struct { Key string Val string } func main() { // Create test data entries := make([]Entry, 1000) for i := 0; i < 1000; i++ { entries[i].Key = fmt.Sprintf("k%03d", i) entries[i].Val = fmt.Sprintf("v%03d", i) } // Test different encoding/compression techniques for _, name := range []string{"Naked", "flate", "zlib", "gzip", "bzip2"} { buf := &bytes.Buffer{} var out io.Writer switch name { case "Naked": out = buf case "flate": out, _ = flate.NewWriter(buf, flate.DefaultCompression) case "zlib": out, _ = zlib.NewWriterLevel(buf, zlib.DefaultCompression) case "gzip": out = gzip.NewWriter(buf) case "bzip2": out, _ = bzip2.NewWriter(buf, nil) } enc := gob.NewEncoder(out) for _, e := range entries { enc.Encode(e) } if c, ok := out.(io.Closer); ok { c.Close() } fmt.Printf("[%5s] Length: %5d, average: %5.2f / Entry\n", name, buf.Len(), float64(buf.Len())/1000) } }
Output:
[Naked] Length: 16053, average: 16.05 / Entry [flate] Length: 3988, average: 3.99 / Entry [ zlib] Length: 3994, average: 3.99 / Entry [ gzip] Length: 4006, average: 4.01 / Entry [bzip2] Length: 1977, average: 1.98 / Entry
As evident from the output, using compression techniques significantly reduces the file size, with bzip2 achieving an impressive 1.98 bytes/Entry.
The above is the detailed content of How Can I Efficiently Serialize Go Structs to Disk with Minimal File Size?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










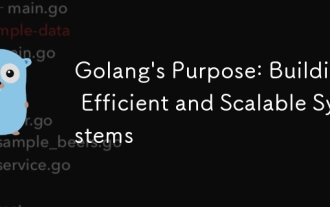
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
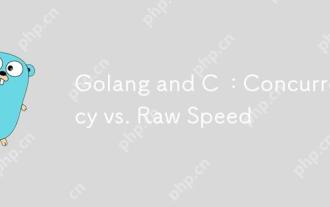
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
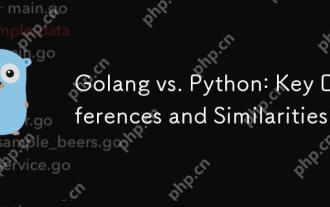
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
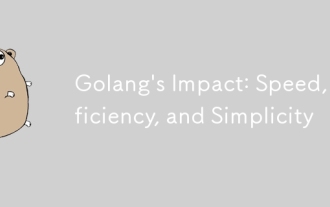
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
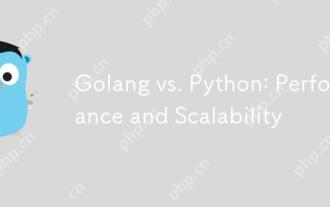
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
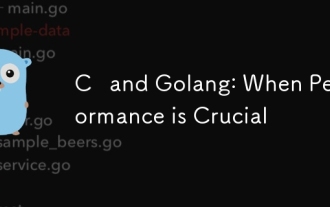
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
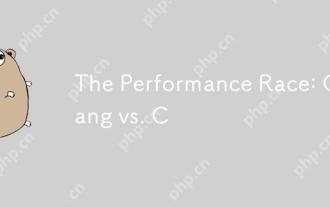
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
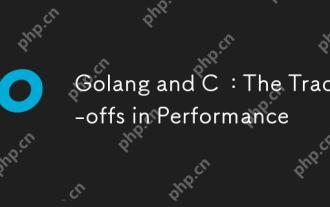
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
