How Can We Achieve Type-Safe Discriminated Unions in C#?
Discriminated Union in C
Introduction:
Discriminated unions, also known as tagged unions or disjoint unions, are a programming construct that allows the storage of different types of data in a single variable. They provide a type-safe way to represent a set of values with a limited number of variants.
Understanding the Problem:
The question proposes a Union class in C# to provide similar functionality to the C-style union. However, the class lacks compiler-enforced type checking when using the Is and As functions. The issue arises when attempting to retrieve a value of a specific type without ensuring that the union actually contains that type.
Can We Achieve Type-Safe Union in C#?
Yes, we can implement type-safe discriminated unions in C# using generic constraints and pattern matching. Here's an example:
public abstract class Union<A, B, C> { public abstract T Match<T>(Func<A, T> f, Func<B, T> g, Func<C, T> h); } public class Case1<A, B, C> : Union<A, B, C> { public readonly A Item; public Case1(A item) { Item = item; } public override T Match<T>(Func<A, T> f, Func<B, T> g, Func<C, T> h) => f(Item); } public class Case2<A, B, C> : Union<A, B, C> { public readonly B Item; public Case2(B item) { Item = item; } public override T Match<T>(Func<A, T> f, Func<B, T> g, Func<C, T> h) => g(Item); } public class Case3<A, B, C> : Union<A, B, C> { public readonly C Item; public Case3(C item) { Item = item; } public override T Match<T>(Func<A, T> f, Func<B, T> g, Func<C, T> h) => h(Item); }
Usage:
To use this Union type, create instances of specific cases:
var union1 = new Case1<int, string, bool>(5); var union2 = new Case2<int, string, bool>('a'); var union3 = new Case3<int, string, bool>(true);
Pattern Matching:
To retrieve the value from the union, use pattern matching:
var value1 = union1.Match(n => n, _ => null, _ => null); // Returns 5 (int) var value2 = union2.Match(_ => null, c => c.ToString(), _ => null); // Returns "a" (string) var value3 = union3.Match(_ => null, _ => null, b => b.ToString()); // Returns "True" (string)
Conclusion:
This solution provides type-safe discriminated unions in C# by leveraging generics and pattern matching. It ensures that the code does not attempt to access incorrect values, enhancing the safety and correctness of the application.
The above is the detailed content of How Can We Achieve Type-Safe Discriminated Unions in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










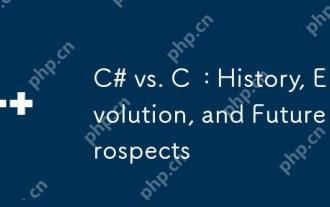
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
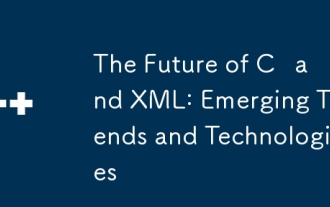
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
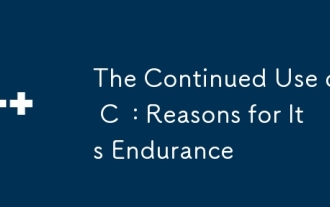
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
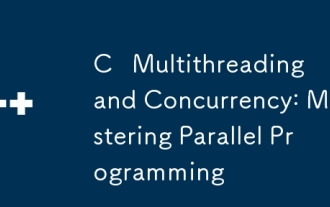
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
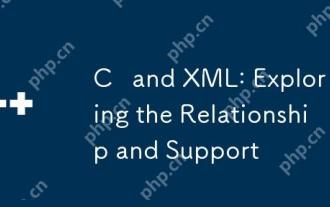
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
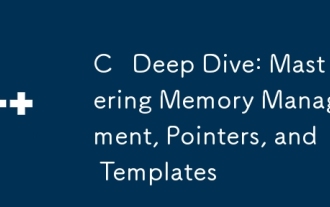
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
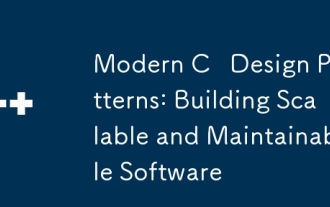
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
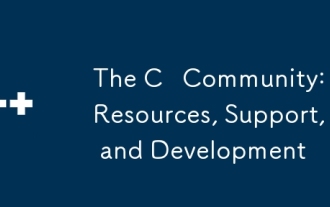
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
