


Why Does FastAPI Execute API Calls Serially Instead of in Parallel?
Jan 02, 2025 pm 03:15 PMFastAPI: Why API Calls Are Executed Serially Instead of in Parallel
FastAPI defines endpoints (also known as path operation functions) using both async def and def. While conceptually async def may suggest parallelization, FastAPI actually handles these functions differently:
Endpoints Defined with async def:
- Run directly in the event loop.
- Can only be called from other async functions and must pause (await) before executing non-async operations, such as I/O.
- Ensure the event loop is not blocked and that other tasks can be executed concurrently.
Endpoints Defined with def:
- Run not directly in the event loop but instead in a separate thread from an external threadpool.
- Can be called from either async or non-async functions.
- May block the event loop and prevent other tasks from executing if non-async operations are performed without pausing.
- Offer performance optimizations in certain scenarios.
Impact on Parallelization:
Based on this understanding, let's examine your code example:
1 2 3 4 5 6 |
|
In this case, the following occurs:
- Two requests to /ping are sent simultaneously.
- The async defENDPOINT runs directly in the event loop.
- The time.sleep(5) call pauses the event loop for 5 seconds.
- During these 5 seconds, the second request is queued and cannot be processed since the event loop is blocked.
- Once the event loop resumes after 5 seconds, the second request is processed.
As a result, the responses are printed serially:
1 2 3 4 |
|
To enable parallelization, non-async operations like time.sleep() should not be used in async def endpoints. Instead, one of the following approaches can be applied:
- Use run_in_threadpool() to spawn a thread and execute the blocking operation outside the event loop.
- Use loop.run_in_executor() or asyncio.to_thread() to run the blocking operation in a separate thread or process.
- Consider using ThreadPoolExecutor or ProcessPoolExecutor to run computationally intensive tasks off-process.
The above is the detailed content of Why Does FastAPI Execute API Calls Serially Instead of in Parallel?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
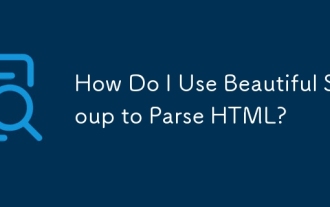
How Do I Use Beautiful Soup to Parse HTML?
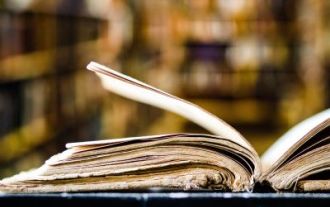
How to Use Python to Find the Zipf Distribution of a Text File
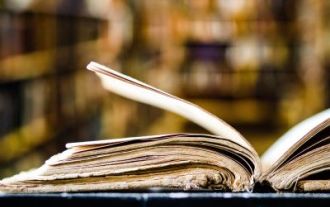
How to Work With PDF Documents Using Python
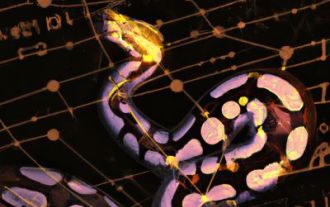
How to Cache Using Redis in Django Applications
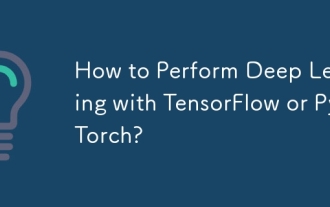
How to Perform Deep Learning with TensorFlow or PyTorch?
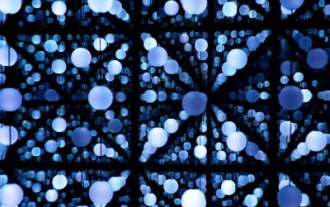
How to Implement Your Own Data Structure in Python
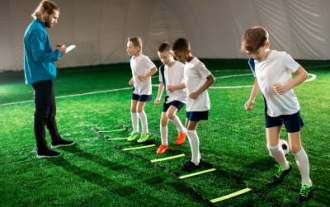
Introduction to Parallel and Concurrent Programming in Python
