


Why Does Direct Slice Conversion in Go Reuse the Same Memory Address for Pointers?
Go Slices and Memory Reusability
A curious issue arose in a project involving Go pointers. The problem was that when converting a slice of struct objects to a slice of interfaces, the first pointer's memory address was repeatedly used in the output.
To resolve this issue, the developer modified the conversion function to use an extra variable, which yielded the expected output.
This raises the question: why did the original solution fail? To understand this, we need to delve into how Go handles pointers and slices.
In Go, the expression *coll returns a slice header containing information about the underlying array, its length, and its capacity. When accessing an element of a slice, the expression (*coll)[idx] is used, which returns a reference to the element at index idx.
In the original solution, item was the loop variable in the range *coll loop. This loop iterates over the slice header, assigning each element of the slice to the loop variable item. However, since item is the loop variable, its memory address remains the same throughout the loop. Therefore, when &item is appended to the output slice, the same memory address is added multiple times, resulting in the observed behavior.
The revised solution uses the expression i := (*coll)[idx] within the loop to assign the element at index idx to a local variable i. This variable has a distinct memory address from the loop variable item, and thus, when &i is added to the output slice, each element has a different memory address.
To illustrate the difference in memory addresses between the loop variable and the element being accessed, consider the following code:
package main import "fmt" func main() { coll := []int{5, 10, 15} for i, v := range coll { fmt.Printf("This one is always the same; %v\n", &v) fmt.Println("This one is 4 bytes larger each iteration; %v\n", &coll[i]) } }
Running this code will demonstrate that &v has the same memory address for all iterations of the loop, while &coll[i] has a different memory address for each iteration.
The above is the detailed content of Why Does Direct Slice Conversion in Go Reuse the Same Memory Address for Pointers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
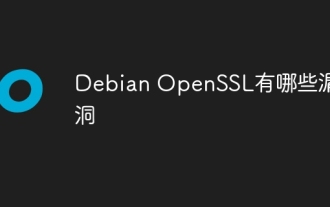
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
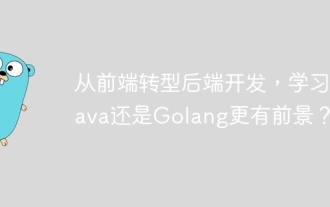
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
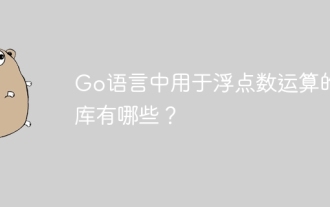
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
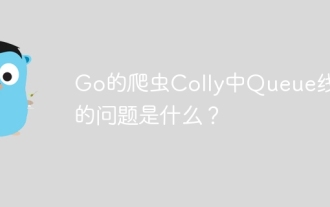
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
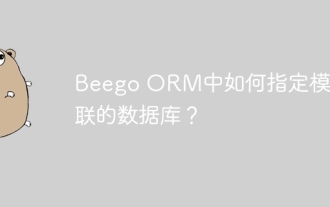
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
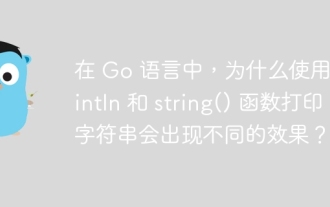
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
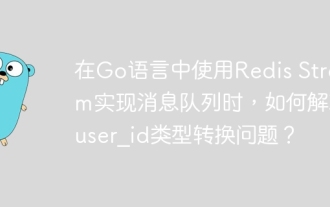
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
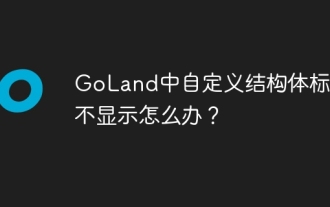
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
