How to Ensure Valid User Input in Python?
Asking for Valid User Input Until Received
When requesting user input, it's crucial to handle invalid responses gracefully rather than crashing or accepting incorrect values. The following techniques ensure valid input is obtained:
Try/Except for Exceptional Input
Use try/except to catch specific input that cannot be parsed. For instance:
while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Sorry, that's not a valid age.") continue break
Custom Validation for Additional Rules
Sometimes, input that can be parsed may still not meet certain criteria. You can add custom validation logic to reject specific values:
while True: data = input("Enter a positive number: ") if int(data) < 0: print("Invalid input. Please enter a positive number.") continue break
Combining Exception Handling and Custom Validation
Combine both techniques to handle both invalid parsing and custom validation rules:
while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Sorry, that's not a valid age.") continue if age < 0: print("Invalid age. Please enter a positive number.") continue break
Encapsulation in a Function
To reuse the custom input validation logic, encapsulate it in a function:
def get_positive_age(): while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Sorry, that's not a valid age.") continue if age < 0: print("Invalid age. Please enter a positive number.") continue return age
Advanced Input Sanitization
You can create a more generic input function to handle various validation scenarios:
def get_valid_input(prompt, type_=None, min_=None, max_=None, range_=None): while True: try: value = type_(input(prompt)) except ValueError: print(f"Invalid input type. Expected {type_.__name__}.") continue if max_ is not None and value > max_: print(f"Value must be less than or equal to {max_}.") continue if min_ is not None and value < min_: print(f"Value must be greater than or equal to {min_}.") continue if range_ is not None and value not in range_: template = "Value must be {}." if len(range_) == 1: print(template.format(*range_)) else: expected = " or ".join(( ", ".join(str(x) for x in range_[:-1]), str(range_[-1]) )) print(template.format(expected)) else: return value
This function enables you to specify the data type, range, and other constraints for the user input.
The above is the detailed content of How to Ensure Valid User Input in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










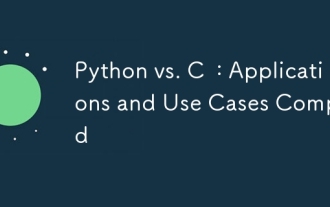
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
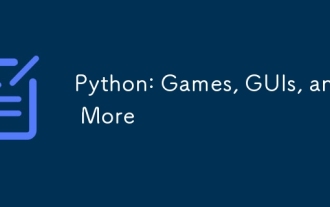
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
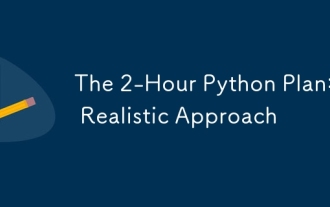
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
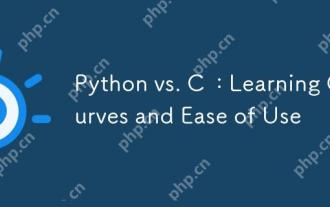
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
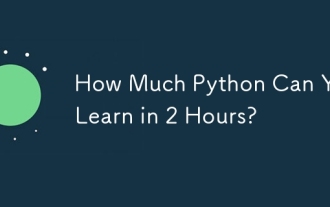
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
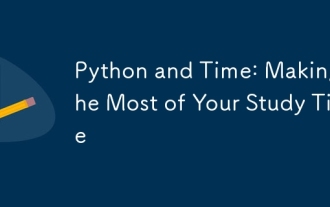
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
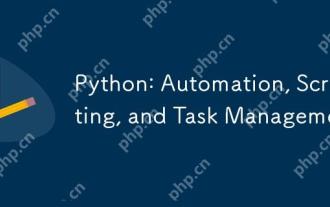
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
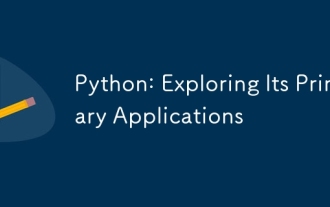
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
