How to Sort Alphanumeric Strings Correctly in C#?
Sorting Strings Alphanumerically
In programming, it is often necessary to sort data based on specific criteria. One common scenario is sorting strings that may contain both alphabetic and numeric characters. However, sorting such strings naively using the default ordering may not yield the desired results.
Consider the following code snippet:
string[] things = new string[] { "105", "101", "102", "103", "90" }; foreach (var thing in things.OrderBy(x => x)) { Console.WriteLine(thing); }
This code aims to sort an array of strings numerically,但得到的输出却是不正确的:
101, 102, 103, 105, 90
Instead of sorting the strings based on their numeric value, the default ordering treats them as strings and sorts them alphabetically, resulting in the output above.
Custom Comparer to Handle Numeric Strings
To address this issue, we can define a custom comparer that can handle both alphabetic and numeric strings. The idea is to use Enumerable.OrderBy, which allows us to specify a custom comparer to control the sorting behavior.
Here is how we can implement a custom comparer using the IComparer
public class SemiNumericComparer : IComparer<string> { public int Compare(string s1, string s2) { const int S1GreaterThanS2 = 1; const int S2GreaterThanS1 = -1; var IsNumeric1 = IsNumeric(s1); var IsNumeric2 = IsNumeric(s2); if (IsNumeric1 && IsNumeric2) { var i1 = Convert.ToInt32(s1); var i2 = Convert.ToInt32(s2); if (i1 > i2) { return S1GreaterThanS2; } if (i1 < i2) { return S2GreaterThanS1; } return 0; } if (IsNumeric1) { return S2GreaterThanS1; } if (IsNumeric2) { return S1GreaterThanS2; } return string.Compare(s1, s2, true, CultureInfo.InvariantCulture); } public static bool IsNumeric(string value) { return int.TryParse(value, out _); } }
This comparer checks if both strings are numeric and sorts them accordingly. If only one string is numeric, it prioritizes the alphabetic string.
To use the custom comparer, we can modify the code as follows:
foreach (var thing in things.OrderBy(x => x, new SemiNumericComparer())) { Console.WriteLine(thing); }
Now, the output will be correctly sorted both alphabetically and numerically:
007, 90, bob, lauren, paul
By using a custom comparer, we can effectively sort strings that contain numeric characters without needing to convert them to integers, addressing the initial challenge and achieving the desired sorting behavior.
The above is the detailed content of How to Sort Alphanumeric Strings Correctly in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


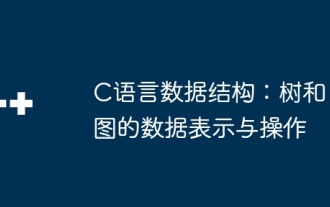
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
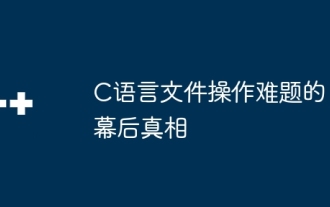
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
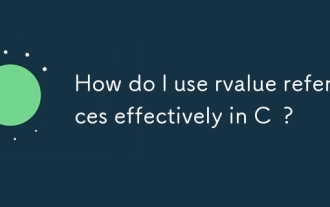
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
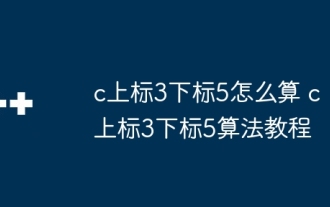
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
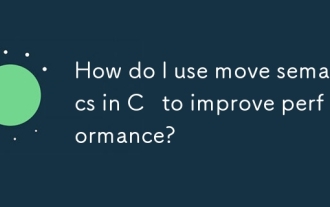
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
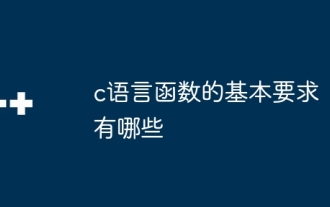
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
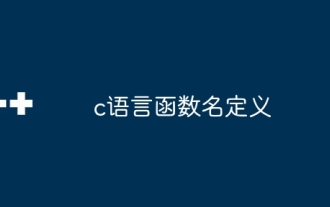
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
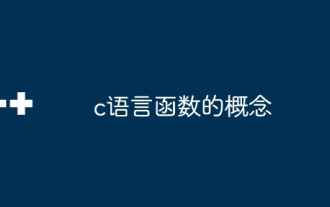
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
