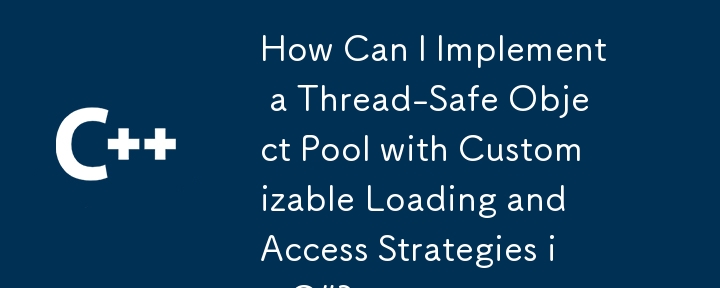
Object Pooling
Problem: Implementing a shared object pool strategy for limited resource management, similar to SQL connection pooling, with thread-safe access.
Answers:
1. Resource Loading Strategy:
-
Eager: Preloads all resources at pool creation.
-
Lazy: Creates resources only when needed.
-
Lazy Expanding: Creates resources up to the pool size limit.
2. Access Strategy:
-
FIFO (First-In-First-Out): Resources are accessed in the order they are created.
-
LIFO (Last-In-First-Out): Resources are accessed in reverse order of creation.
-
Circular Buffer: Resources are accessed in a circular order.
3. Implementation Details:
- Utilizes a "round robin" approach using a circular buffer to approximate round-robin access.
- Different interface implementations handle LIFO, FIFO, and circular access patterns.
4. Loading and Access Modes:
- Loading modes: Eager, Lazy, LazyExpanding
- Access modes: FIFO, LIFO, Circular
Advantages:
- Provides a thread-safe, general-purpose resource pool.
- Offers flexible configurations for different loading and access strategies.
- Allows resources to be reused and shared across multiple callers.
Usage Example:
To use the object pool, create an instance of the Pool class with desired settings. Then, use the Acquire() method to obtain a resource from the pool and the Release() method to return it.
Conclusion:
This implementation provides a robust and configurable object pooling mechanism that can be adapted to various resource management scenarios in C#.
The above is the detailed content of How Can I Implement a Thread-Safe Object Pool with Customizable Loading and Access Strategies in C#?. For more information, please follow other related articles on the PHP Chinese website!