


How Can I Reuse a Single Postgres DB Connection in Go for Efficient Row Inserts?
Reusing a Single Postgres DB Connection in Go for Row Inserts
In the realm of database operations, one of the common tasks is inserting rows into a table. For optimal database performance, it is essential to establish a single connection and reuse it for all insert operations. This approach avoids the overhead of opening numerous connections and ensures efficient database interactions.
Issue: Opening Excessive DB Connections
When attempting to insert multiple rows into a Postgres table using Go, it is crucial to avoid opening a new connection for each insert operation. If your code resembles the following:
db, err := sql.Open("postgres", "connectionURL") if err != nil { log.Fatal(err) } for i := 0; i < 10; i++ { // Insert row _, err := db.Exec("INSERT INTO table VALUES (...)") if err != nil { log.Fatal(err) } }
You will encounter the issue of opening multiple connections. This is because the db variable is a connection pool rather than a single connection. Each time you call Exec, a new connection is opened and added to the pool.
Solution: Reusing a Single Connection
To ensure that a single connection is used for multiple inserts, it is necessary to make the following modifications:
- Define a global *sql.DB variable outside the main function and initialize it in the init function. This ensures that the connection is established only once during program execution.
- In the main function, where the actual insert operations are performed, no new connections should be opened. Instead, the global db variable should be used for all subsequent insert operations.
The corrected code should resemble the following:
var db *sql.DB func init() { var err error db, err = sql.Open("postgres", "connectionURL") if err != nil { log.Fatal(err) } if err = db.Ping(); err != nil { log.Fatal(err) } } func main() { for i := 0; i < 10; i++ { // Insert row _, err := db.Exec("INSERT INTO table VALUES (...)") if err != nil { log.Fatal(err) } } }
By implementing these changes, you can effectively reuse a single Postgres DB connection for all insert operations, eliminating the issue of opening excessive connections and maximizing database efficiency.
The above is the detailed content of How Can I Reuse a Single Postgres DB Connection in Go for Efficient Row Inserts?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










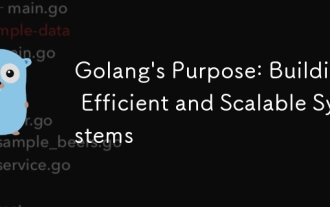
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
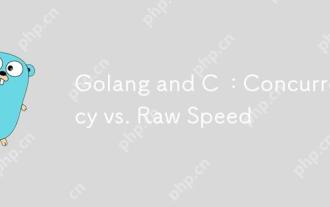
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
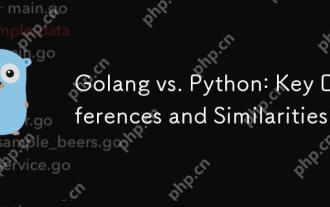
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
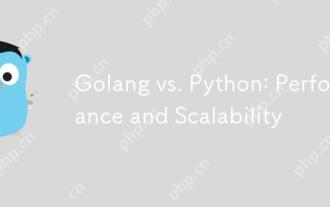
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
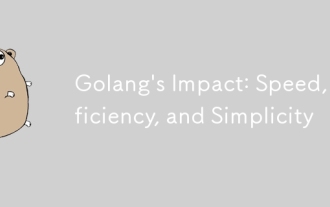
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
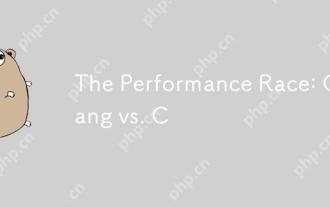
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
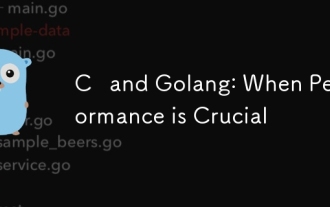
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
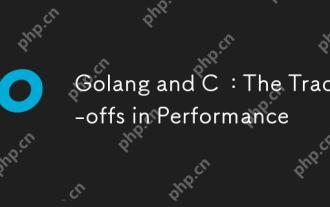
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
