How Can I Parse HTTP Requests and Responses from a Text File in Go?
Parse HTTP Requests and Responses from Text File in Go
In Go, parsing HTTP requests and responses from a text file involves leveraging the built-in HTTP parsing functions. To achieve this, one can employ the following approach:
func ReadHTTPFromFile(r io.Reader) ([]Connection, error) { // Establish a new buffered reader to process the input. buf := bufio.NewReader(r) // Define a slice that will store HTTP connection information. stream := make([]Connection, 0) // Loop indefinitely to parse request and response pairs until we reach the end of the file. for { // Attempt to parse an HTTP request using ReadRequest. req, err := http.ReadRequest(buf) // Check if we have reached the end of the file or an error occurred. if err == io.EOF { // Break out of the loop since we have reached the end of the file. break } else if err != nil { // Log the error and return the partially parsed stream. log.Println("Error parsing HTTP request:", err) return stream, err } // Now that we have a request, we need to parse the corresponding HTTP response. resp, err := http.ReadResponse(buf, req) // Check for any errors while parsing the response. if err != nil { // Log the error and return the partially parsed stream. log.Println("Error parsing HTTP response:", err) return stream, err } // Copy the response body to a new buffer to preserve it. var b bytes.Buffer io.Copy(&b, resp.Body) // Close the original response body and replace it with a new, non-closing one. resp.Body.Close() resp.Body = ioutil.NopCloser(&b) // Add the connection to our stream. stream = append(stream, Connection{Request: req, Response: resp}) } // Return the parsed stream. return stream, nil }
With this function, you can open a file containing HTTP requests and responses and parse them. For example:
func main() { // Open a file for reading. file, err := os.Open("http.txt") if err != nil { log.Fatal(err) } // Parse the HTTP requests and responses from the file. stream, err := ReadHTTPFromFile(file) if err != nil { log.Fatal(err) } // Dump a representation of the parsed requests and responses for inspection. for _, c := range stream { reqDump, err := httputil.DumpRequest(c.Request, true) if err != nil { log.Fatal(err) } respDump, err := httputil.DumpResponse(c.Response, true) if err != nil { log.Fatal(err) } fmt.Println(string(reqDump)) fmt.Println(string(respDump)) } }
This code will read the contents of the "http.txt" file, parse the HTTP requests and responses, and dump their representation for inspection. The HTTP parsing functions provided by the Go standard library enable you to extract and manipulate requests and responses from a text file stream.
The above is the detailed content of How Can I Parse HTTP Requests and Responses from a Text File in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
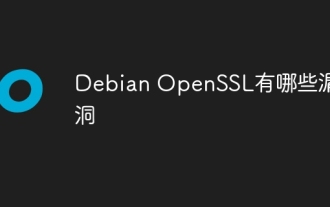
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
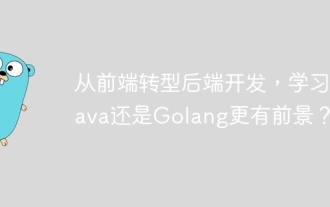
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
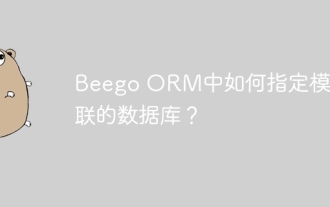
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
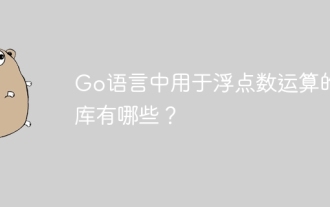
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
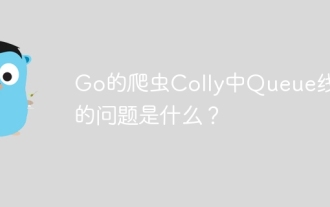
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
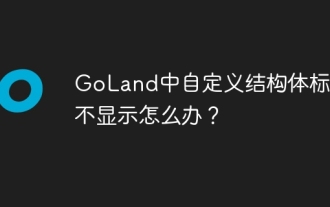
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
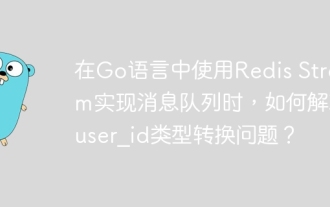
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...

This article introduces how to configure MongoDB on Debian system to achieve automatic expansion. The main steps include setting up the MongoDB replica set and disk space monitoring. 1. MongoDB installation First, make sure that MongoDB is installed on the Debian system. Install using the following command: sudoaptupdatesudoaptinstall-ymongodb-org 2. Configuring MongoDB replica set MongoDB replica set ensures high availability and data redundancy, which is the basis for achieving automatic capacity expansion. Start MongoDB service: sudosystemctlstartmongodsudosys
