


How Can I Safely Parse an Empty String or Invalid Input to an Integer in C#?
Jan 03, 2025 pm 06:44 PMHandling Empty Input for Integer Parsing
When attempting to parse an empty string using int.Parse, you may encounter the error: "Input string was not in a correct format." This occurs because an empty string does not represent a valid integer.
Alternative Approaches
To address this issue, several options are available:
1. Default to 0 on Empty Input (with Exception for Invalid Formats):
int i = string.IsNullOrEmpty(Textbox1.Text) ? 0 : int.Parse(Textbox1.Text);
This code checks if the text is empty and, if so, assigns 0 to the i variable. Otherwise, it parses the text to an integer as usual. However, any non-integer input will still cause an exception.
2. Default to 0 with Any Invalid Input:
int i; if (!int.TryParse(Textbox1.Text, out i)) i = 0;
This approach uses int.TryParse to attempt to parse the text to an integer. If successful, the result is assigned to the i variable. If the text is empty or contains non-integer data, i is set to 0. This method tolerates all invalid input without throwing an exception.
The above is the detailed content of How Can I Safely Parse an Empty String or Invalid Input to an Integer in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
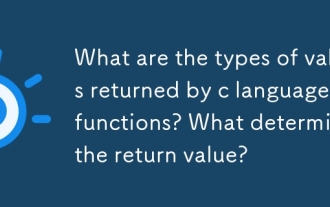
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
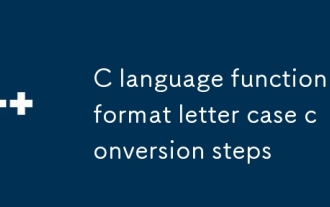
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
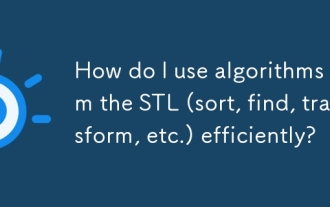
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
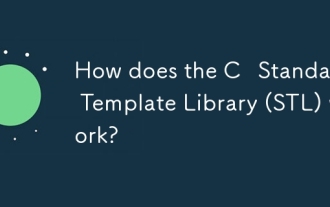
How does the C Standard Template Library (STL) work?
