How Can I Connect and Use SQLite Databases with Java?
Java and SQLite: A Guide to Connecting and Using
In the pursuit of a more efficient and compact database solution, SQLite has emerged as a popular choice. This article explores the options available for connecting and utilizing SQLite with the Java programming language.
The Convenient Choice: JavaSQLite
One prominent library for interfacing Java with SQLite is JavaSQLite. This lightweight wrapper provides an intuitive API that simplifies database interactions. By simply adding the JavaSQLite JAR file to your project's classpath, you can connect to SQLite databases and perform various operations.
A Direct Solution: JDBC
For a more direct approach, consider using the Java JDBC driver for SQLite. This driver allows you to interact with SQLite databases using the familiar JDBC API. You can add the sqlitejdbc-v056.jar to your classpath and import the necessary java.sql.* packages.
A Practical Example
To demonstrate the ease of use with the JDBC driver, consider this Java code snippet:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.Statement; public class Test { public static void main(String[] args) throws Exception { Class.forName("org.sqlite.JDBC"); Connection conn = DriverManager.getConnection("jdbc:sqlite:test.db"); Statement stat = conn.createStatement(); ... } }
This code illustrates how to connect to a SQLite database, create a table, insert data, and perform a query. The test.db database file will be created in the project's root directory, providing a simple and convenient way to work with a local database.
Additional Resources
For more information on Java and SQLite integration, consider the following resources:
- [JavaSQLite Documentation](https://www.ch-werner.de/javasqlite)
- [SQLite JDBC Driver](https://bitbucket.org/xerial/sqlite-jdbc)
- [JDBC Tutorial](https://docs.oracle.com/javase/tutorial/jdbc/)
The above is the detailed content of How Can I Connect and Use SQLite Databases with Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










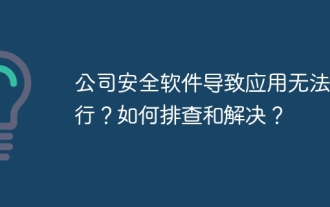
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
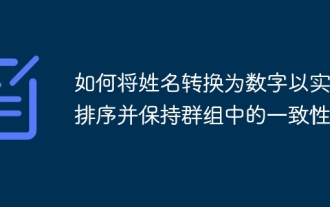
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
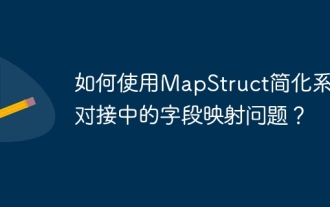
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
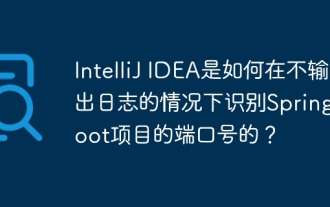
Start Spring using IntelliJIDEAUltimate version...
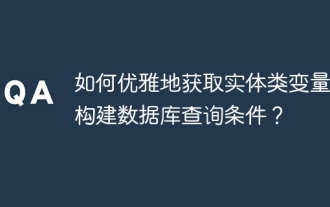
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
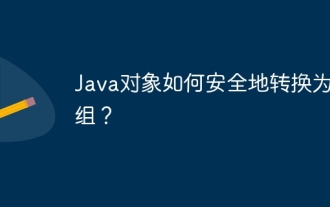
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
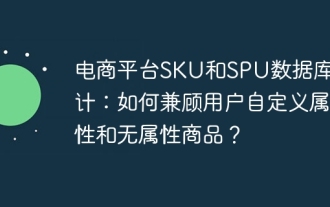
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
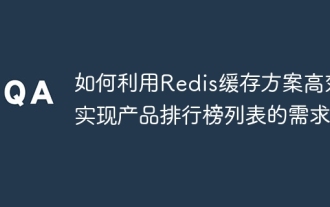
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
