


WSGI vs ASGI: The Crucial Decision Shaping Your Web App's Future in 5
WSGI and ASGI are two gateway interfaces designed for Python, acting as a communication bridge between web servers and web applications. With the evolving demands of modern web applications, these two protocols have established their distinct characteristics and use cases.
What is a Gateway Interface (GI)?
A gateway interface is a communication protocol between a web server and a web application. It standardizes interactions to enable the execution of dynamic scripts while ensuring compatibility across different implementations.
Common gateway interface protocols include:
- CGI (Common Gateway Interface)
- FastCGI (Fast Common Gateway Interface)
- WSGI (Web Server Gateway Interface)
- ASGI (Asynchronous Server Gateway Interface)
WSGI: A Closer Look
Background and Characteristics
WSGI (Web Server Gateway Interface) is a standard interface defined in PEP 3333 for communication between Python web applications and web servers. Its synchronous and blocking design makes it well-suited for handling HTTP-based synchronous requests.
WSGI was created to simplify the interaction between web servers and Python applications, addressing compatibility issues across frameworks and servers, and enabling easier development of web applications.
Example WSGI Code
# wsgi_app.py def simple_app(environ, start_response): status = '200 OK' headers = [('Content-type', 'text/plain')] start_response(status, headers) return [b"Hello, WSGI World!"] if __name__ == "__main__": from wsgiref.simple_server import make_server server = make_server('localhost', 8080, simple_app) print("Serving on port 8080...") server.serve_forever()
Explanation:
-
simple_app is a WSGI-compliant application function with two parameters:
- environ: A dictionary containing HTTP request data.
- start_response: A callback function for setting response headers and status.
- The application runs on a local server and returns "Hello, WSGI World!".
Popular Frameworks Using WSGI
- Django: A full-featured and mature web framework.
- Flask: A lightweight framework ideal for small projects or microservices.
ASGI: A Modern Evolution
Background and Characteristics
With the introduction of async and await in Python 3.5 , asynchronous programming became increasingly popular. However, WSGI’s synchronous design couldn't leverage these capabilities.
ASGI (Asynchronous Server Gateway Interface) was developed to fill this gap. Initially proposed by the Django Channels project, ASGI supports modern protocols like WebSocket and HTTP/2, making it suitable for real-time communication and high-concurrency scenarios.
Key Features of ASGI:
- Asynchronous Non-blocking: Handles large numbers of concurrent requests effectively.
- Protocol Versatility: Supports HTTP, WebSocket, HTTP/2, and more.
- High Scalability: Perfect for real-time communication and complex workloads.
Example ASGI Code
# wsgi_app.py def simple_app(environ, start_response): status = '200 OK' headers = [('Content-type', 'text/plain')] start_response(status, headers) return [b"Hello, WSGI World!"] if __name__ == "__main__": from wsgiref.simple_server import make_server server = make_server('localhost', 8080, simple_app) print("Serving on port 8080...") server.serve_forever()
Explanation:
-
app is an ASGI-compliant function with three parameters:
- scope: A dictionary with information about the request, such as protocol type and path.
- receive: An asynchronous function for receiving client messages.
- send: An asynchronous function for sending responses.
- The application uses Uvicorn as the ASGI server to serve requests.
Popular Frameworks Using ASGI
- FastAPI: A modern, high-performance web framework for building APIs with Python.
WSGI vs. ASGI: Key Differences
Feature | WSGI | ASGI | |||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
Synchronous, Blocking | Asynchronous, Non-blocking | |||||||||||||||
Concurrency Handling | Limited | Excellent | |||||||||||||||
Protocol Support | HTTP Only | HTTP, WebSocket, HTTP/2 | |||||||||||||||
Use Case | Traditional Applications | Real-time, High-concurrency Apps |
Conclusion: How to Choose?
The choice between WSGI and ASGI depends on your specific use case:
- Choose WSGI: For traditional web applications like blogs or corporate websites that rely on HTTP and don’t require high concurrency or real-time communication.
- Choose ASGI: For applications requiring real-time communication (e.g., chat apps) or handling high-concurrency workloads with protocols like WebSocket or HTTP/2.
Leapcell: The Ideal Platform for WSGI and ASGI Applications:
Leapcell is a cloud computing platform designed for modern distributed applications. Its pay-as-you-go pricing ensures no idle costs—users only pay for the resources they use.
Unique Advantages of Leapcell for WSGI/ASGI Applications:
1. Multi-Language Support
- Develop with JavaScript, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
2. Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
3. Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
4. Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the documentation!
Leapcell Twitter: https://x.com/LeapcellHQ
The above is the detailed content of WSGI vs ASGI: The Crucial Decision Shaping Your Web App's Future in 5. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
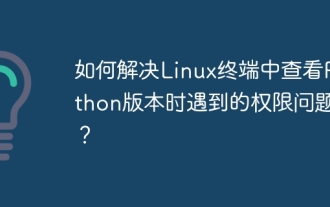
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
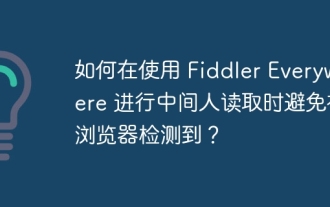
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
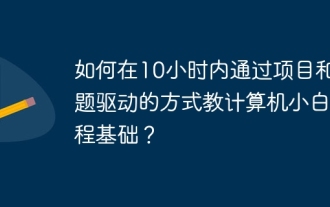
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
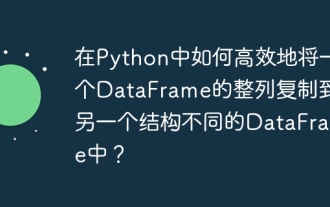
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
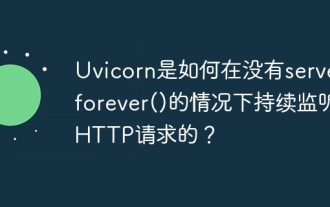
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
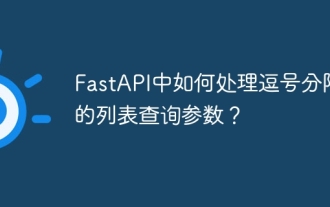
Fastapi ...
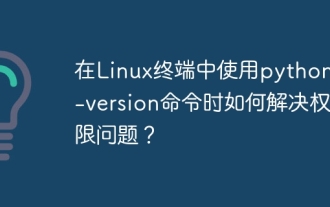
Using python in Linux terminal...
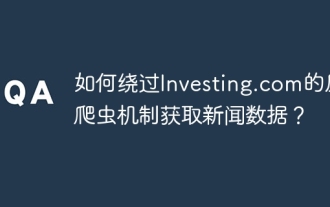
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
