How to Properly Deep Copy a List in Python?
How to Deep Copy a List?
When attempting to create a deep copy of a list, it's crucial to avoid using the list() constructor. While list() may appear to generate a distinct list, it only performs a shallow copy, preserving references to the original list's elements. Consequently, any modifications made to the new list will also affect the original.
Solution: Deep Copy Using copy.deepcopy()
For true deep copying, the copy.deepcopy() function must be employed. This function recursively clones all elements within the list, ensuring that the new list is independent of the original.
Example:
import copy # Original list E0 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] # Shallow copy E0_copy1 = list(E0) # Deep copy E0_copy2 = copy.deepcopy(E0) # Modify shallow copy E0_copy1[0][0] = 0 # Observe that changes to the shallow copy also affect the original print(E0) # Output: [[0, 2, 3], [4, 5, 6], [7, 8, 9]] # Modify deep copy E0_copy2[1][1] = 0 # Note that changes to the deep copy do not affect the original print(E0) # Output: [[0, 2, 3], [4, 5, 6], [7, 8, 9]]
Explanation:
list() initializes a new list by referencing the original elements. Therefore, any alterations made to the copy propagates to the original list.
copy.deepcopy(), on the other hand, creates copies of all nested elements within the list, resulting in a fully independent copy. Modifications to the deep copy do not impact the original list.
The above is the detailed content of How to Properly Deep Copy a List in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










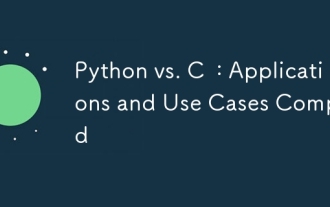
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
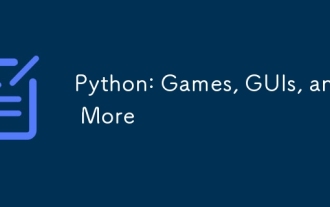
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
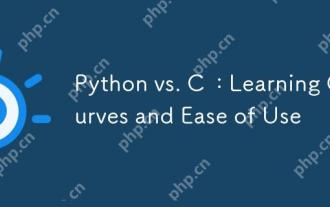
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
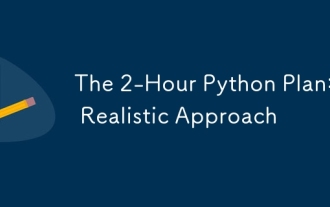
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
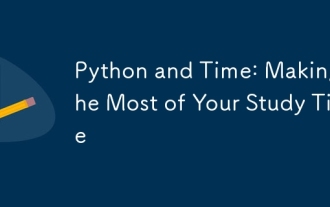
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
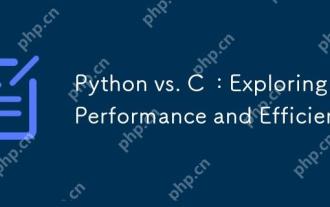
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
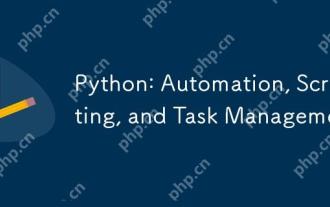
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
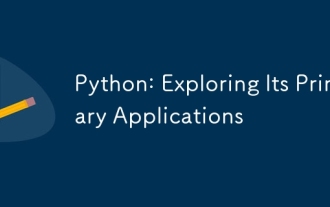
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
