How to Extract SQL Code from Entity Framework Core IQueryable?
Extract SQL Code from Entity Framework Core IQueryable
In Entity Framework Core, obtaining the SQL code generated by an IQueryable query is crucial for debugging and performance analysis. However, unlike previous versions of Entity Framework, ToTraceString is no longer available in EF Core.
EF Core 5/6
In recent versions of EF Core (5.0 and above), you can retrieve the SQL code using the ToQueryString() method:
var query = _context.Widgets.Where(w => w.IsReal && w.Id == 42); var sql = query.ToQueryString();
EF Core 2.1.2 and Older
For older versions of EF Core (2.1.2 and below), you can use the following code to obtain the SQL code:
public static string ToSql<TEntity>(this IQueryable<TEntity> query) { // ... (code omitted for brevity) ... }
Note that this code requires an extension method definition to access internal fields of EF Core.
EF Core 3.0
In EF Core 3.0, you can use the following method:
public static string ToSql<TEntity>(this IQueryable<TEntity> query) { // ... (code omitted for brevity) ... }
This method also requires an extension method definition to access internal fields.
EF Core 3.1 and Above
Starting from EF Core 3.1, you can use the following method:
public static string ToSql<TEntity>(this IQueryable<TEntity> query) where TEntity : class { // ... (code omitted for brevity) ... }
This method uses reflection to access internal fields and provide SQL code from IQueryable queries.
The EF Core team also plans to introduce an official way to obtain SQL code in future releases.
The above is the detailed content of How to Extract SQL Code from Entity Framework Core IQueryable?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


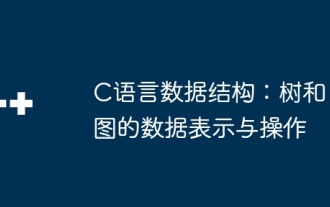
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
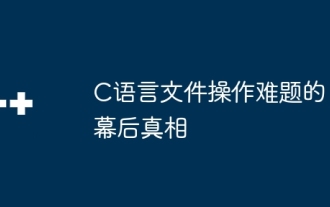
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
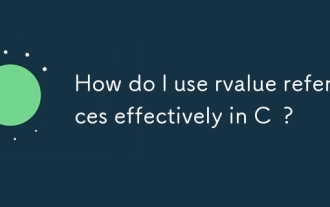
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
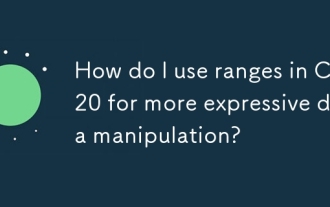
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
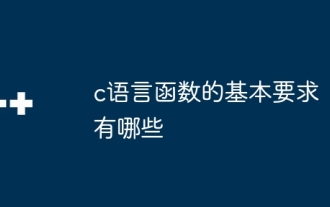
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
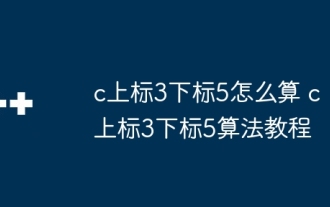
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
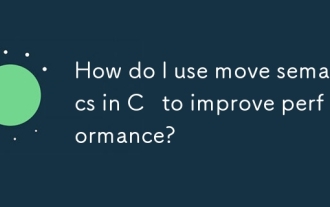
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
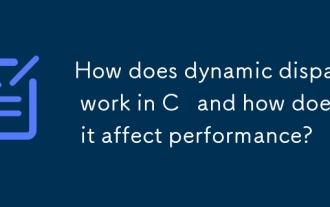
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
