


How Can Python's `natsort` Library Perform Natural String Sorting?
Jan 04, 2025 am 07:10 AMPerforming Natural Sorting with Strings in Python
In Python, performing a natural alphabetical sort on a list of strings can be achieved using third-party libraries. One such library is natsort, which provides a variety of methods for natural sorting.
For instance, to sort a list of strings in a natural order, one can use natsorted():
from natsort import natsorted list = ['Elm11', 'Elm12', 'Elm2', 'elm0', 'elm1', 'elm10', 'elm13', 'elm9'] natsorted(list, key=lambda y: y.lower()) # or natsorted(list, alg=ns.IGNORECASE) # Output: ['elm0', 'elm1', 'Elm2', 'elm9', 'elm10', 'Elm11', 'Elm12', 'elm13']
Alternatively, a sorting key can be generated using natsort_keygen():
from natsort import natsort_keygen natsort_key = natsort_keygen(key=lambda y: y.lower()) # or natsort_keygen(alg=ns.IGNORECASE) list.sort(key=natsort_key) # Output: ['elm0', 'elm1', 'Elm2', 'elm9', 'elm10', 'Elm11', 'Elm12', 'elm13']
The natsort library utilizes a generalized algorithm to handle various types of input, including strings with special characters and numbers. Its documentation provides detailed explanations and examples of its sorting capabilities.
In addition, natsort now includes os_sorted to sort paths in a manner consistent with the operating system's file system browser.
from natsort import os_sorted os_sorted(list_of_paths) # Sorts paths like the file system browser
The above is the detailed content of How Can Python's `natsort` Library Perform Natural String Sorting?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
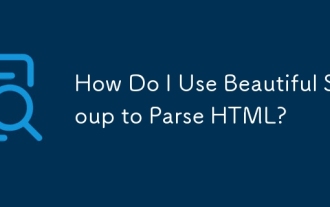
How Do I Use Beautiful Soup to Parse HTML?
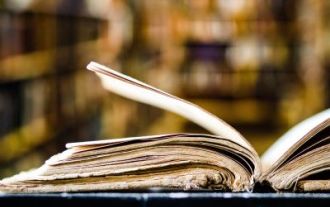
How to Use Python to Find the Zipf Distribution of a Text File
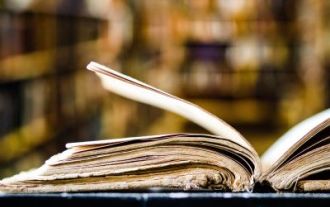
How to Work With PDF Documents Using Python
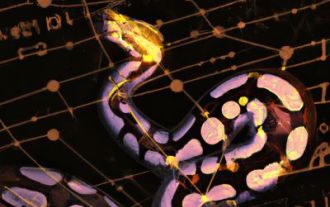
How to Cache Using Redis in Django Applications
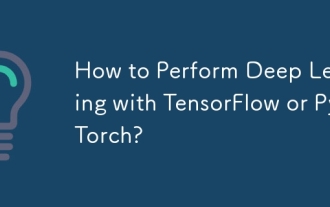
How to Perform Deep Learning with TensorFlow or PyTorch?
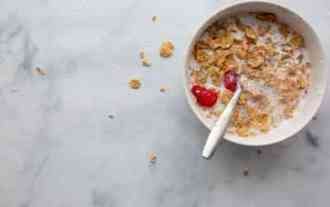
Serialization and Deserialization of Python Objects: Part 1
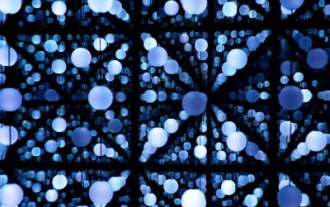
How to Implement Your Own Data Structure in Python
