How Do I Assert Exception Handling in JUnit Tests?
Asserting Exception Handling in JUnit Tests
In JUnit, testing code that should throw an exception requires a clean and concise approach. While manually checking for exceptions is possible, it's not the idiomatic way.
JUnit 5 and 4.13
In JUnit versions 5 and 4.13, you can use @Test(expected = ExceptionClass.class) annotation on the test method. This expects the specified exception to be thrown.
Example:
@Test(expected = IndexOutOfBoundsException.class) public void testIndexOutOfBoundsException() { ArrayList emptyList = new ArrayList(); emptyList.get(0); }
AssertJ and Google-Truth
If using libraries like AssertJ or Google-Truth, you can employ their assertions for validating exceptions.
AssertJ:
import static org.assertj.core.api.Assertions.assertThatThrownBy; @Test public void testFooThrowsIndexOutOfBoundsException() { assertThatThrownBy(() -> foo.doStuff()).isInstanceOf(IndexOutOfBoundsException.class); }
Google-Truth:
import static com.google.common.truth.Truth.assertThat; @Test public void testFooThrowsIndexOutOfBoundsException() { assertThat(assertThrows(IndexOutOfBoundsException.class, foo::doStuff)).isNotNull(); }
JUnit <= 4.12
In JUnit versions less than or equal to 4.12, you can use Rule or TryCatch to handle exceptions.
Using Rule:
@Rule public ExpectedException thrown = ExpectedException.none(); @Test public void testIndexOutOfBoundsException() { thrown.expect(IndexOutOfBoundsException.class); ArrayList emptyList = new ArrayList(); emptyList.get(0); }
Using TryCatch:
import static org.junit.Assert.assertEquals; @Test public void testIndexOutOfBoundsException() { try { ArrayList emptyList = new ArrayList(); emptyList.get(0); fail("IndexOutOfBoundsException was expected"); } catch (IndexOutOfBoundsException e) { assertEquals(e.getClass(), IndexOutOfBoundsException.class); } }
The above is the detailed content of How Do I Assert Exception Handling in JUnit Tests?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










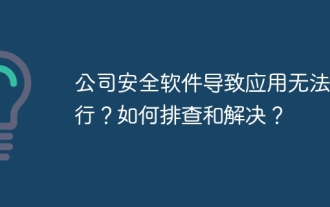
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
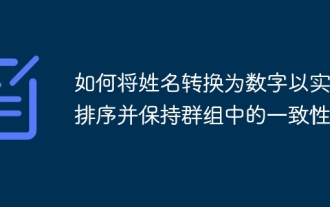
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
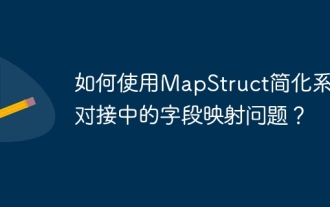
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
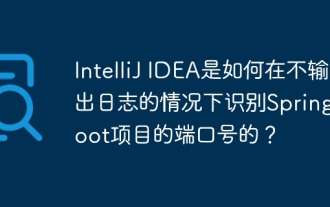
Start Spring using IntelliJIDEAUltimate version...
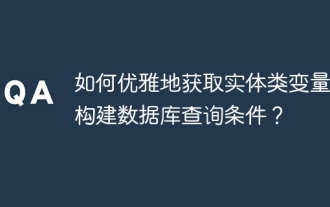
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
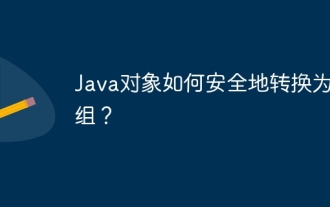
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
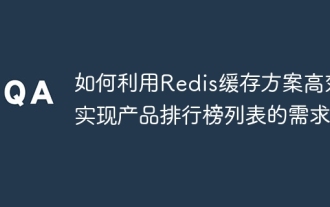
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
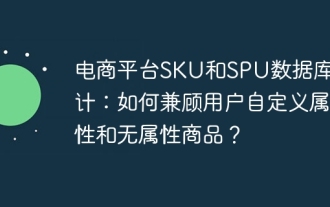
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
