How to Accurately Convert UTC to Local Time in Go?
How to Convert UTC to Local Time in Go
Problem Statement
Converting UTC time to local time can be a challenge, especially when considering time zone variations. In Go, some users have encountered incorrect results when attempting to add UTC time offset durations to the current time.
Solution
The issue lies in the handling of time zones. While adding the time offset duration can adjust the time value, it does not account for the specific time zone's rules and DST (Daylight Saving Time) changes. To accurately convert UTC to local time, the proper way is to use the time.LoadLocation function.
Code Example
The following code demonstrates how to use time.LoadLocation to obtain local time in specific locations:
var countryTz = map[string]string{ "Hungary": "Europe/Budapest", "Egypt": "Africa/Cairo", } func timeIn(name string) time.Time { loc, err := time.LoadLocation(countryTz[name]) if err != nil { panic(err) } return time.Now().In(loc) } func main() { utc := time.Now().UTC().Format("15:04") hun := timeIn("Hungary").Format("15:04") eg := timeIn("Egypt").Format("15:04") fmt.Println(utc, hun, eg) }
In this example, countryTz defines a map of countries and their corresponding time zone names. The timeIn function loads the appropriate time zone and converts the current time to the local time in that time zone.
By using time.LoadLocation, the code ensures that time zone rules and DST adjustments are taken into account, providing accurate local times.
The above is the detailed content of How to Accurately Convert UTC to Local Time in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
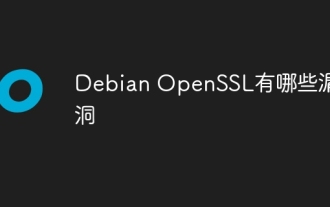
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
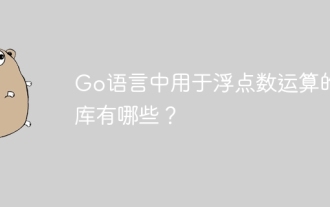
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
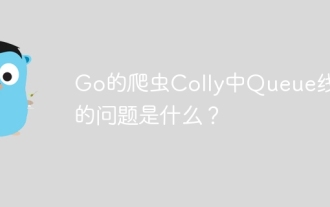
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
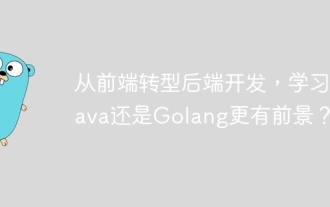
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
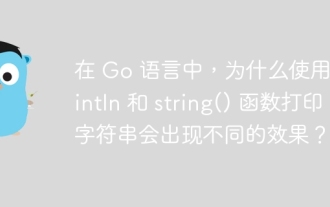
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
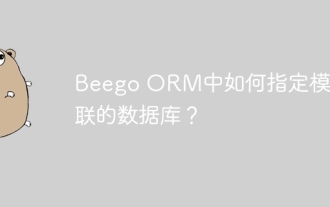
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
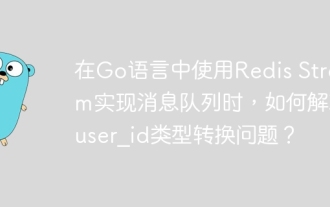
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
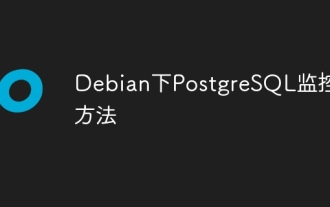
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
