How to Seamlessly Combine Images in C#/.NET?
Combining Images Seamlessly in C#/.NET
Enhancing an image or creating a visually compelling composition often involves merging separate images. In C#, this process is straightforward, using the powerful classes and objects available in the .NET Framework.
Consider the task of merging two images: a transparent 500x500 image and a 150x150 image. The goal is to create a new image where the transparent section of the larger image allows the smaller image to appear beneath it.
To accomplish this in C#, utilize the following steps:
- Load the Images: Load both images into their corresponding Image objects using the Image.FromFile() method.
- Create a Blank Canvas: Create a new Bitmap object with the desired size (500x500) to serve as the canvas for the merged image.
- Draw the Larger Image: Using the Graphics.FromImage() method, create a Graphics object associated with the canvas. Draw the larger image (500x500) onto the canvas using the DrawImage() method.
- Draw the Smaller Image: Position the smaller image (150x150) in the center of the canvas using the provided InterpolationMode for quality. Use the DrawImage() method to draw the smaller image onto the canvas at the desired location.
- Save the Merged Image: Once the two images are drawn onto the canvas, save the resulting image to the desired file location using the Save() method.
Here's a code sample that demonstrates the merging process:
Image playbutton, frame; try { playbutton = Image.FromFile(/*path to smaller image*/); frame = Image.FromFile(/*path to larger image*/); } catch (Exception ex) { return; // handle exceptions gracefully } using (frame) { using (var bitmap = new Bitmap(500, 500)) { using (var canvas = Graphics.FromImage(bitmap)) { // set desired interpolation mode canvas.InterpolationMode = InterpolationMode.HighQualityBicubic; canvas.DrawImage(frame, 0, 0, frame.Width, frame.Height); canvas.DrawImage(playbutton, (bitmap.Width / 2) - (playbutton.Width / 2), (bitmap.Height / 2) - (playbutton.Height / 2)); } try { bitmap.Save(/*desired save path*/, System.Drawing.Imaging.ImageFormat.Jpeg); } catch (Exception ex) { } // handle exceptions gracefully } }
By following these steps and utilizing the provided code sample, you can easily merge images in C#/.NET, enabling you to create visually appealing compositions.
The above is the detailed content of How to Seamlessly Combine Images in C#/.NET?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


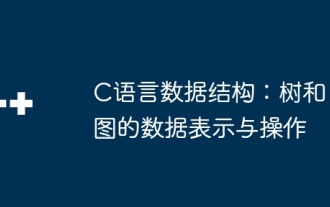
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
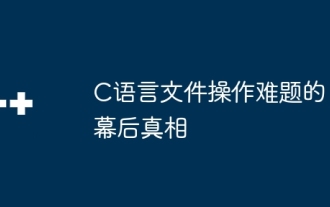
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
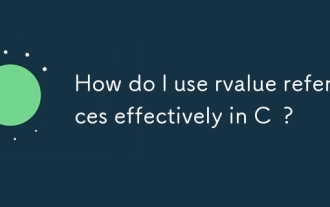
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
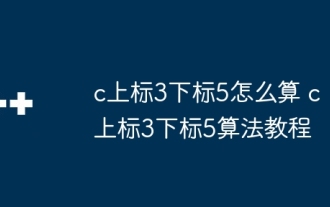
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
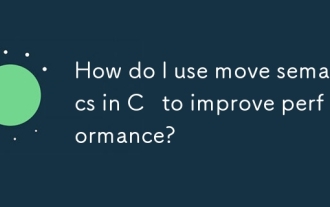
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
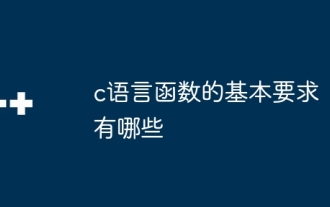
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
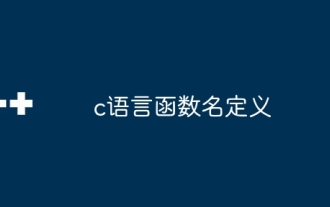
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
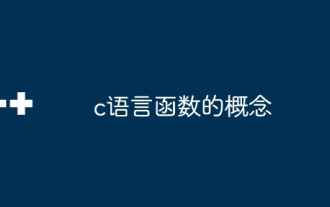
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
