How Can I Create a Textbox with Placeholder Text in C#?
Creating a Textbox with Placeholder Text in C#
It is common practice to provide guidance to users through placeholder text in textboxes. This text appears when the textbox is empty, prompting users to enter the appropriate information. Creating an HTML5 textbox with placeholder text is straightforward, but how can we achieve this in C#?
The TextBox class in C# provides various properties and events that can be utilized to create a similar functionality. Here's how you can add placeholder text to a textbox:
Implementation:
Textbox myTxtbx = new Textbox(); myTxtbx.Text = "Enter text here..."; myTxtbx.GotFocus += GotFocus.EventHandle(RemoveText); myTxtbx.LostFocus += LostFocus.EventHandle(AddText); public void RemoveText(object sender, EventArgs e) { if (myTxtbx.Text == "Enter text here...") { myTxtbx.Text = ""; } } public void AddText(object sender, EventArgs e) { if (string.IsNullOrWhiteSpace(myTxtbx.Text)) myTxtbx.Text = "Enter text here..."; }
Explanation:
- Initialization: We create a new TextBox named 'myTxtbx' and set its initial text to the placeholder text.
- Event Handlers: The 'GotFocus' event is triggered when the textbox receives focus, and the 'LostFocus' event is triggered when focus is lost. These events will be used to add and remove the placeholder text.
- Remove Text: The RemoveText method removes the placeholder text when the user clicks on the textbox. It checks if the current text is the placeholder text and replaces it with an empty string.
- Add Text: The AddText method adds the placeholder text when the textbox loses focus and there is no user-entered text. It checks if the textbox text is empty or whitespace and updates it with the placeholder text.
This approach simulates the placeholder text behavior seen in HTML5 by adding and removing the placeholder text based on focus events. It provides a convenient and user-friendly way to guide users when interacting with text input fields in C#.
The above is the detailed content of How Can I Create a Textbox with Placeholder Text in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










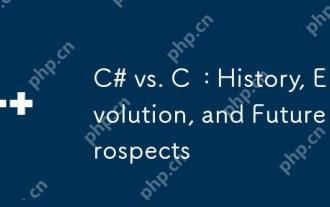
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
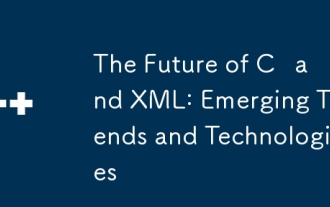
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
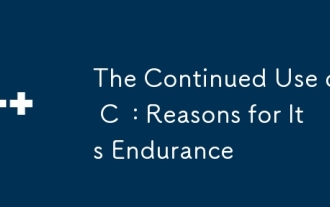
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
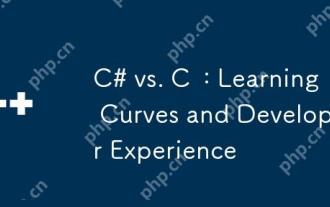
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
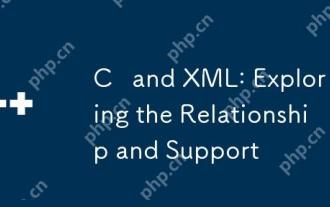
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
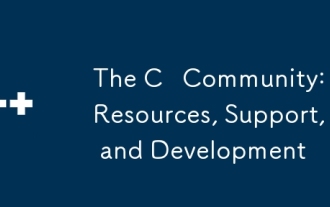
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
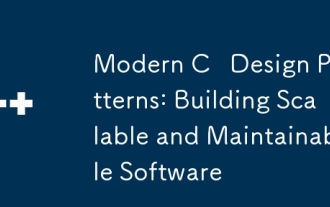
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
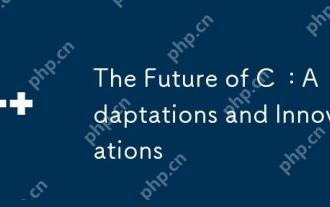
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
