


How Can I Extract Node Attribute Values Using Python's ElementTree?
Extracting Node Attribute Values with Python
In an XML document, it's often necessary to retrieve instances of a particular node attribute. Consider the following XML structure:
<foo> <bar> <type foobar="1"/> <type foobar="2"/> </bar> </foo>
The goal is to access the values of the attribute foobar, which in this example are "1" and "2".
Using Python's ElementTree library provides an efficient and straightforward solution:
import xml.etree.ElementTree as ET # Parse the XML string or file into an Element instance root = ET.parse('thefile.xml').getroot() # Iterate through 'bar' tags and extract 'foobar' attribute values for type_tag in root.findall('bar/type'): value = type_tag.get('foobar') print(value)
This code effectively accesses and prints the desired attribute values, resulting in the following output:
1 2
ElementTree offers a convenient and powerful API for parsing and manipulating XML data, enabling efficient retrieval of node attribute instances.
The above is the detailed content of How Can I Extract Node Attribute Values Using Python's ElementTree?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
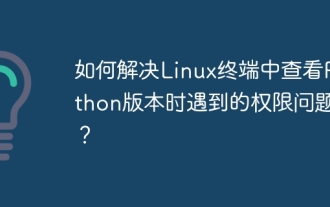
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
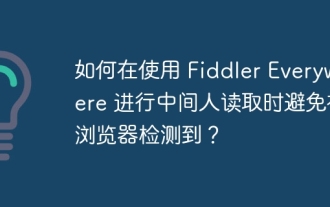
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
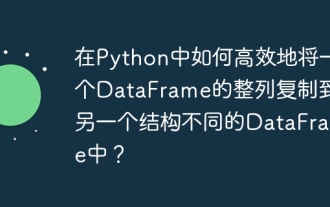
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
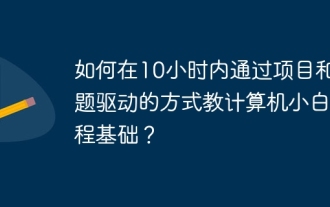
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
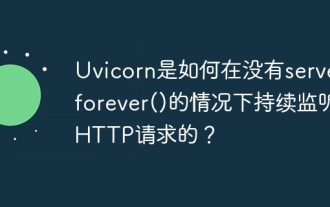
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
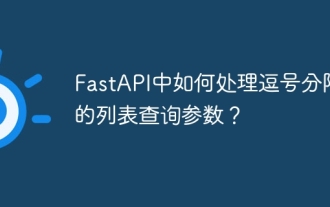
Fastapi ...
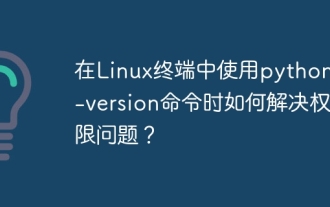
Using python in Linux terminal...
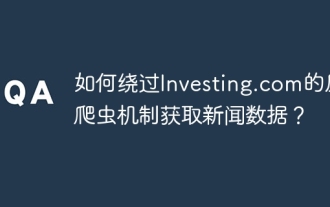
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
