How to Insert Items into JavaScript Arrays Effectively?
How to Effectively Insert Items into Arrays in JavaScript
Inserting items at specific indices in arrays is a common programming task. JavaScript provides several methods to achieve this, including the built-in splice function.
The JavaScript Insert Method: Using splice
The splice method offers a versatile way to insert items into arrays. Its syntax is:
arr.splice(index, deleteCount, item1, item2, ...)
- index: The position where the item should be inserted.
- deleteCount: The number of items to remove before inserting (usually 0).
- item1, item2, ...: The items to insert.
Insertion Example
To insert an item into an array at index 2, you would use the following syntax:
arr.splice(2, 0, item);
JavaScript Implementation in jQuery
While JavaScript natively supports the splice method, jQuery also provides an extension method called insertAt. To use it, you would write:
$(arr).insertAt(index, item);
Example Usage
Consider the following array:
var arr = ['Jani', 'Hege', 'Stale', 'Kai Jim', 'Borge'];
To insert "Lene" at index 2 using the splice method:
arr.splice(2, 0, "Lene");
Console output:
['Jani', 'Hege', 'Lene', 'Stale', 'Kai Jim', 'Borge']
The above is the detailed content of How to Insert Items into JavaScript Arrays Effectively?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


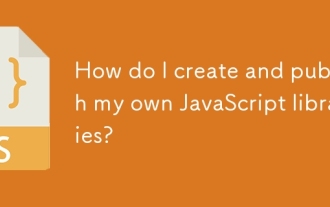
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
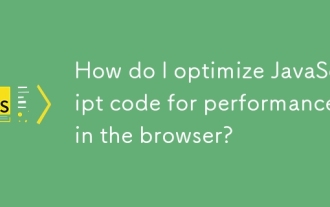
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
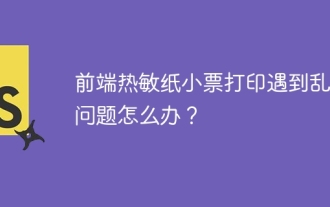
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
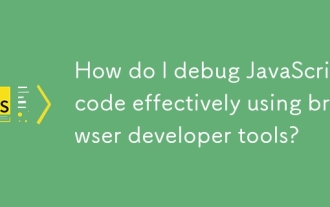
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
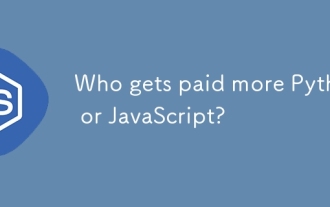
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
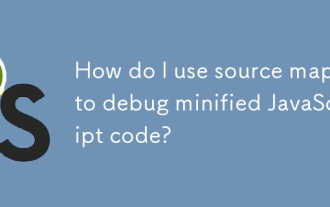
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
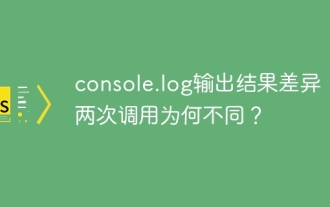
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
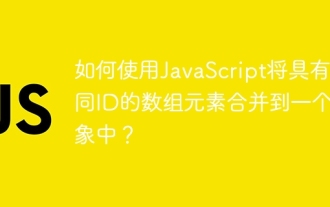
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
