


How can I merge two images in C#/.NET, centering a smaller image over a larger one while preserving transparency?
Merging Images in C#/.NET: A Comprehensive Guide
Introduction
Creating captivating visuals by combining multiple images is a common task in various domains, from image editing to web design. In C#/.NET, this merging process involves utilizing the powerful Graphics API and its associated classes.
Problem Statement
Suppose you have two images: a transparent 500x500 image (ImageA) and a 150x150 image (ImageB). Your goal is to merge these images, positioning ImageB in the center of ImageA while preserving the transparency of ImageA's middle region.
Solution
The solution begins by creating an empty canvas of size 500x500. Subsequently, you draw ImageB onto the canvas, aligning it centrally. Finally, you draw ImageA over the canvas, allowing its transparent center to reveal ImageB.
Implementation
The following C# code provides a detailed implementation of this merging process:
using System.Drawing; namespace ImageMerger { public static class Program { public static void Main(string[] args) { // Load the images Image imageA = Image.FromFile("path/to/imageA.png"); Image imageB = Image.FromFile("path/to/imageB.png"); // Create an empty canvas int width = imageA.Width; int height = imageA.Height; using (var bitmap = new Bitmap(width, height)) { // Draw the base image onto the canvas using (var canvas = Graphics.FromImage(bitmap)) { canvas.InterpolationMode = InterpolationMode.HighQualityBicubic; canvas.DrawImage(imageA,new Rectangle(0,0,width,height),new Rectangle(0,0,imageA.Width,imageA.Height),GraphicsUnit.Pixel); // Calculate the position of the overlay image int x = (width - imageB.Width) / 2; int y = (height - imageB.Height) / 2; // Draw the overlay image onto the canvas canvas.DrawImage(imageB, x, y); } // Save the merged image to a file bitmap.Save("path/to/mergedImage.png", ImageFormat.Png); } } } }
In this code, the Graphics class provides the necessary methods for drawing the images onto the canvas. The InterpolationMode property ensures high-quality image resampling when scaling the images. The Bitmap class encapsulates the canvas and allows you to save the merged image to a file.
Conclusion
By utilizing the Graphics API and its associated classes, merging images in C#/.NET becomes a straightforward task. The code snippet provided in this article demonstrates how to effectively combine transparent and non-transparent images, creating dynamic and engaging visuals for various applications.
The above is the detailed content of How can I merge two images in C#/.NET, centering a smaller image over a larger one while preserving transparency?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


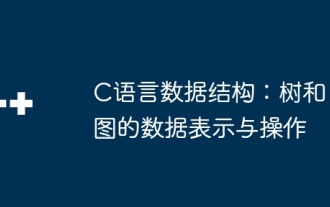
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
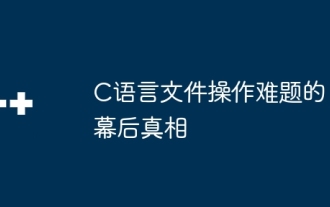
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
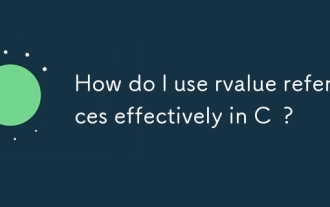
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
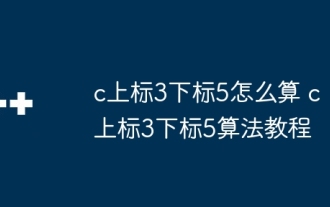
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
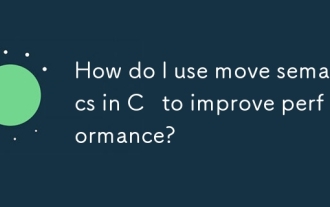
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
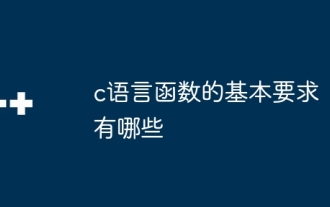
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
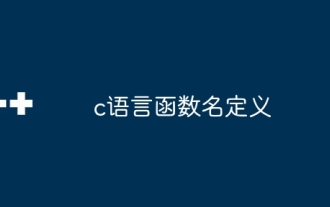
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
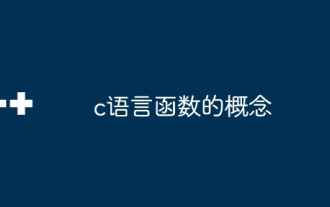
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
