


Return vs. Print: When Should You Use Each for Function Output?
Function Output Handling: Return vs. Print
In programming, handling the output of a function is crucial for efficient code design. While printing and returning functions offer different purposes, they can easily be misunderstood.
Printing:
Printing output using the print() function simply displays the result in the console. It does not store or provide access to the output beyond the context of the function call.
Returning:
When a function returns a value, it stores the output in a variable within the scope of the function. This variable can be accessed and manipulated further, allowing the function's result to be integrated into a larger program.
Differences:
The key difference lies in the availability of the output after the function call. Printed output is only visible during the function's execution and is not available outside of that scope. Returned values, on the other hand, persist in the variable and can be accessed throughout the program's execution.
Consider the example function autoparts():
def autoparts(): parts_dict = {} list_of_parts = open('list_of_parts.txt', 'r') for line in list_of_parts: k, v = line.split() parts_dict[k] = v print(parts_dict)
If autoparts() is called and not assigned to a variable, it will print the parts dictionary but immediately discard it after the function call ends. However, if we modify the function to return the dictionary:
def autoparts(): parts_dict = {} list_of_parts = open('list_of_parts.txt', 'r') for line in list_of_parts: k, v = line.split() parts_dict[k] = v return parts_dict
We can now access the parts dictionary even after the function call, allowing us to further process or store the data.
Conclusion:
Understanding the difference between printing and returning output is essential for writing robust and modular code. While printing provides immediate visual feedback, returning values allows for greater flexibility and control over the function's output.
The above is the detailed content of Return vs. Print: When Should You Use Each for Function Output?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
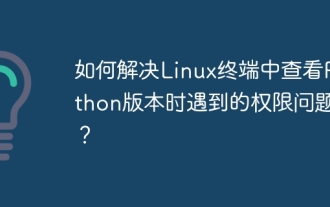
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
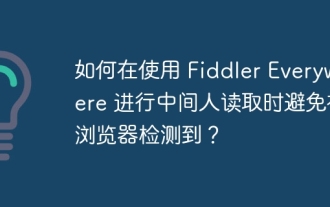
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
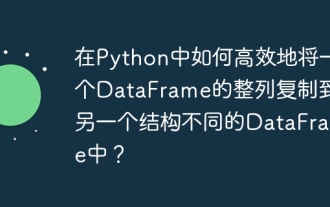
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
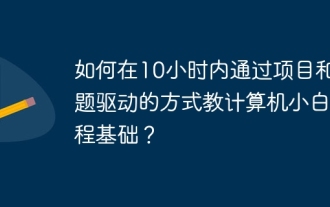
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
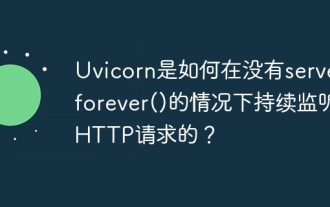
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
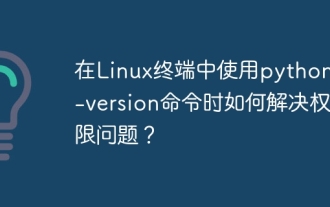
Using python in Linux terminal...
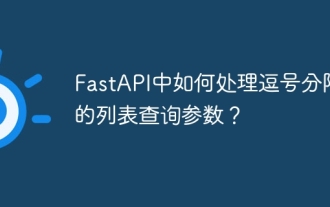
Fastapi ...
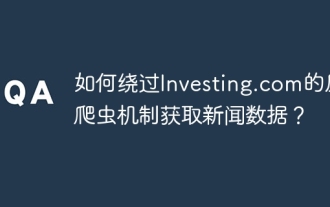
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
