How to Animate a Line Drawing Across a WPF Canvas Using C#?
How to Animate a Line on a Canvas in C
Question: How can I make a line slowly draw across the screen? I want to use C# code in a WPF project.
Answer:
To animate a line on a canvas in C#, follow these steps:
- Create a canvas control on your WPF form.
- Create a LineViewModel class that represents the line you want to animate.
- Bind the LineViewModel to the canvas control.
- In the LineViewModel class, define a Timer that will be used to animate the line.
- In the Timer_Tick event handler, update the coordinates of the line to create the animation effect.
- Set the Animate property to true to start the animation.
Here is an example code snippet that demonstrates how to create and animate a line on a canvas using the provided steps:
XAML:
<Canvas x:Name="MyCanvas" Height="500" Width="500"> <Line x:Name="MyLine" X1="0" Y1="0" X2="100" Y2="100" Stroke="Black" StrokeThickness="2"/> </Canvas>
C# Code:
public partial class MainWindow : Window { private LineViewModel _lineViewModel; public MainWindow() { InitializeComponent(); // Create the LineViewModel. _lineViewModel = new LineViewModel(); // Bind the LineViewModel to the Line control. MyLine.SetBinding(Line.X1Property, new Binding("X1") { Source = _lineViewModel }); MyLine.SetBinding(Line.Y1Property, new Binding("Y1") { Source = _lineViewModel }); MyLine.SetBinding(Line.X2Property, new Binding("X2") { Source = _lineViewModel }); MyLine.SetBinding(Line.Y2Property, new Binding("Y2") { Source = _lineViewModel }); // Start the animation. _lineViewModel.Animate = true; } } public class LineViewModel : INotifyPropertyChanged { #region Timer-based Animation private System.Threading.Timer Timer; private static Random Rnd = new Random(); private bool _animate; public bool Animate { get { return _animate; } set { _animate = value; NotifyPropertyChanged("Animate"); if (value) StartTimer(); else StopTimer(); } } private int _animationSpeed = 1; public int AnimationSpeed { get { return _animationSpeed; } set { _animationSpeed = value; NotifyPropertyChanged("AnimationSpeed"); if (Timer != null) Timer.Change(0, 100 / value); } } private static readonly List<int> _animationSpeeds = new List<int> { 1, 2, 3, 4, 5 }; public List<int> AnimationSpeeds { get { return _animationSpeeds; } } public void StartTimer() { StopTimer(); Timer = new Timer(x => Timer_Tick(), null, 0, 100 / AnimationSpeed); } public void StopTimer() { if (Timer != null) { Timer.Dispose(); Timer = null; } } private void Timer_Tick() { X1 = X1 + Rnd.Next(-2, 3); Y1 = Y1 + Rnd.Next(-2, 3); X2 = X2 + Rnd.Next(-2, 3); Y2 = Y2 + Rnd.Next(-2, 3); } #endregion #region Coordinates private double _x1; public double X1 { get { return _x1; } set { _x1 = value; NotifyPropertyChanged("X1"); } } private double _y1; public double Y1 { get { return _y1; } set { _y1 = value; NotifyPropertyChanged("Y1"); } } private double _x2; public double X2 { get { return _x2; } set { _x2 = value; NotifyPropertyChanged("X2"); } } private double _y2; public double Y2 { get { return _y2; } set { _y2 = value; NotifyPropertyChanged("Y2"); } } #endregion #region Other Properties private string _name; public string Name { get { return _name; } set { _name = value; NotifyPropertyChanged("Name"); } } private double _thickness; public double Thickness { get { return _thickness; } set { _thickness = value; NotifyPropertyChanged("Thickness"); } } public Color Color1 { get; set; } public Color Color2 { get; set; } private double _opacity = 1; public double Opacity { get { return _opacity; } set { _opacity = value; NotifyPropertyChanged("Opacity"); } } #endregion #region INotifyPropertyChanged public event PropertyChangedEventHandler PropertyChanged; private void NotifyPropertyChanged(string propertyName) { Application.Current.Dispatcher.BeginInvoke((Action)(() => { if (PropertyChanged != null) PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); })); } #endregion }
Using this approach, you can create a line that slowly redraws across the canvas at a customizable speed.
The above is the detailed content of How to Animate a Line Drawing Across a WPF Canvas Using C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


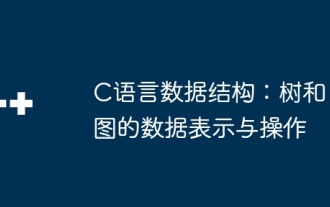
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
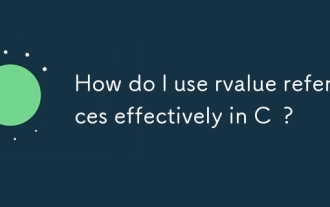
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
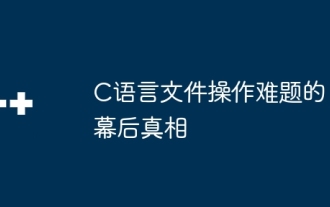
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
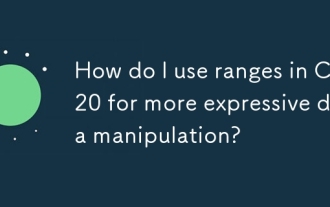
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
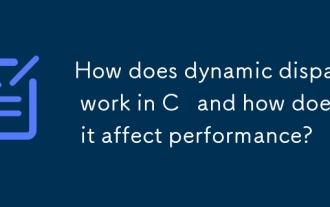
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
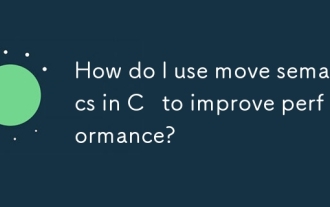
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
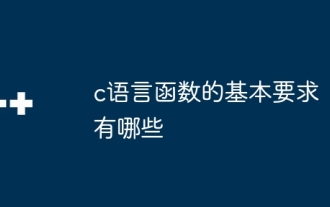
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.

The article discusses auto type deduction in programming, detailing its benefits like reduced code verbosity and improved maintainability, and its limitations such as potential confusion and debugging challenges.
