


How Can I Count and Sort Duplicates Based on a Property in a C# List Using LINQ?
Counting Duplicates Based on Property in a List of Objects with LINQ
In programming, finding duplicates in a list is a common scenario. This article tackles the challenge of counting duplicates based on a specific property within a list of objects using LINQ (Language Integrated Query) in C#.
Suppose you have a list of objects with an ID property, representing a collection of items. Your goal is to count the number of occurrences for each unique ID and sort the results descending by the highest count.
To achieve this, you can leverage LINQ's powerful group by and order by operators. The following steps outline the process:
- Grouping by Property: Utilize the "group by" operation to group the objects in the list by their ID property. This creates sub-groups, where each group contains objects with the same ID.
- Counting Duplicates: Within each group, calculate the count of objects using the "Count" method.
- Sorting by Count: Next, sort the groups by the count of objects in descending order using the "orderby" operator. This arranges the groups based on the highest number of duplicates.
- Selecting Results: Finally, select the desired properties from each group, such as the count, property used for grouping, and any additional information needed.
Code Implementation:
Here's an example code implementation in C#:
var query = list.GroupBy(x => x.ID) .Select(x => new { Count = x.Count(), CategoryID = x.Key, // Other properties as needed }) .OrderByDescending(x => x.Count);
In this example, the "list" represents the collection of objects. The query first groups the objects by their "ID" property, then counts the objects within each group. The resulting anonymous type includes the count and the CategoryID (in this case, the ID of the group). Finally, the results are ordered in descending order based on the count.
This LINQ expression allows you to effortlessly count and sort duplicates based on a specified property in your list of objects.
The above is the detailed content of How Can I Count and Sort Duplicates Based on a Property in a C# List Using LINQ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










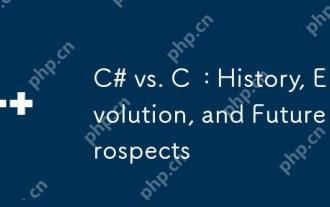
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
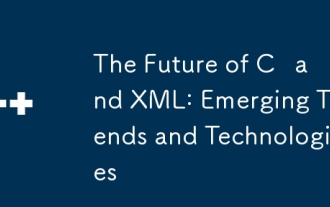
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
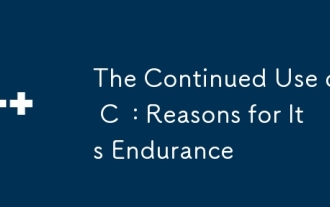
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
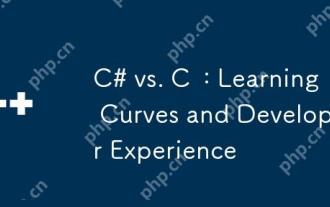
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
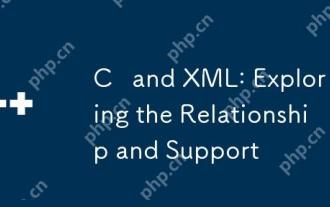
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
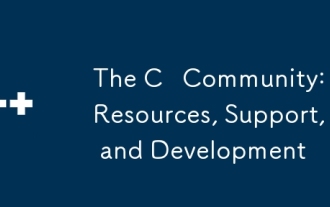
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
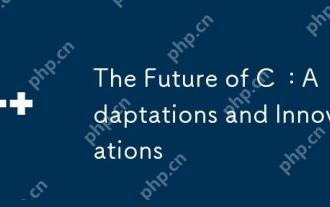
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
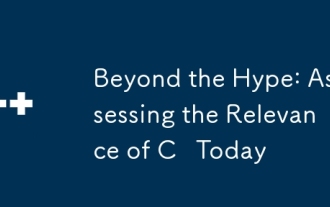
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
