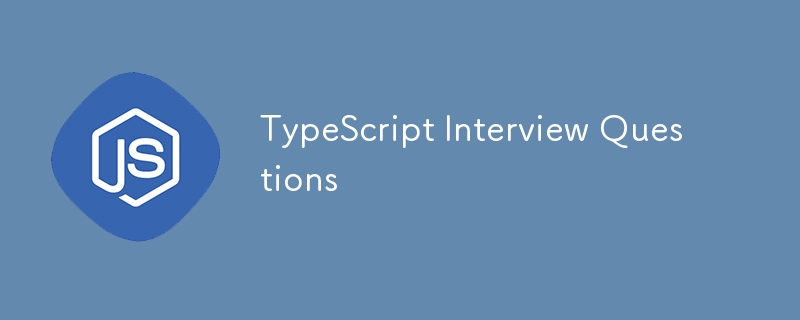
Question - What is TypeScript?
- TypeScript is a superset of Javascript
- Adds static types, allowing for improved code quality and error checking before runtime.
- It supports features like interfaces, enums, generics, and more.
- Provides better error checking, enhanced tools, and improved code readability.
Question - What is explicit and implicit type assignment?
- Explicit means writing out the type. Like below -
let firstName: string = "Rutvik";
Copy after login
Copy after login
Copy after login
- Implicit means TypeScript will guess the type, based on the value. The below type will be considered a number
let age = 23;
Copy after login
Copy after login
Copy after login
Question - Difference between any, unknown and never in TypeScript?
- The type of any is used to assign any type of a variable.
- It will not give error even if you reassign another type.
let x: any = 10;
x = 'hello'; // No TypeScript error
console.log(x.toUpperCase()); // No TypeScript error
Copy after login
Copy after login
Copy after login
- The type unknown is better than type any, because it requires us checking the type before performing operations on value.
let y: unknown = 10;
// Type assertion needed before using y as number
if (typeof y === 'number') {
console.log(y.toFixed(2));
}
Copy after login
Copy after login
Copy after login
- The type never represents value that never occurs.
- It is typically used for return statements of function that doesn’t returns properly.
function throwError(message: string): never {
throw new Error(message);
}
Copy after login
Copy after login
Copy after login
Question - How do you give the type of Arrays?
- For typing array, we need to give the type as below. In this below example the array can contain type of string only.
const names: string[] = ["Rutvik", "Rohit", "Virat"];
names.push("Bumrah"); // no error
Copy after login
Copy after login
Copy after login
- We can also use a readonly keyword, which prevents the array been changed.
const names: readonly string[] = ["Rutvik", "Rohit", "Virat"];
names.push("Bumrah"); // Error: Property 'push' does not exist on type 'readonly string[]'.
Copy after login
Copy after login
Copy after login
Question - What is Type Inference in array?
- If we don’t give any type to an array, it will infer the type automatically.
const numbers = [1, 2, 3]; // inferred to type number[]
numbers.push(4); // no error
Copy after login
Copy after login
Copy after login
Question - What are tuples?
- It is a type array with pre-defined length and types.
- It is very useful in giving types of mixed array with different types.
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
Copy after login
Copy after login
Copy after login
Question - What are readonly tuples?
- If we don’t make a tuple readonly, we can add more items to the one defined and TypeScript will not throw any error.
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
//No safety in indexes from 3
ourTuple.push('This is wrong');
Copy after login
Copy after login
Copy after login
- Now, to fix it we use the keyword readonly before the type.
let ourTuple: readonly [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
// throws error as it is readonly
ourTuple.push('Coding Hero took a day off');
Copy after login
Copy after login
Copy after login
Question - How to give the types for Objects?
- We can give the type of object by creating another object like structure and specifying the keys and the type of the keys in the object.
interface CarTypes {
brand: string,
model: string,
year: number
}
const car: CarTypes = {
brand: "Tata",
model: "Punch",
year: 2020
};
Copy after login
Copy after login
Copy after login
Question - How to have optional properties in Objects?
- To give an optional property or key, we need to add the ? after thet key.
let firstName: string = "Rutvik";
Copy after login
Copy after login
Copy after login
Question - Explain enum in TypeScript?
- An enum is a type of variables which are constants. You have to use the values within it only.
- The values are numeric by default and starts with 0 and increments by 1.
- They can be numeric or string-based
let age = 23;
Copy after login
Copy after login
Copy after login
let x: any = 10;
x = 'hello'; // No TypeScript error
console.log(x.toUpperCase()); // No TypeScript error
Copy after login
Copy after login
Copy after login
Question - What are Type Aliases?
- They allow to define type with a custom name and can be used for all primitive types like string and number and also complex type like objects and arrays.
let y: unknown = 10;
// Type assertion needed before using y as number
if (typeof y === 'number') {
console.log(y.toFixed(2));
}
Copy after login
Copy after login
Copy after login
Question - What are interfaces?
- Interfaces are like type but can be used only for objects.
function throwError(message: string): never {
throw new Error(message);
}
Copy after login
Copy after login
Copy after login
Question - How to extend interfaces?
- Interfaces can be extended with the extend keyword.
const names: string[] = ["Rutvik", "Rohit", "Virat"];
names.push("Bumrah"); // no error
Copy after login
Copy after login
Copy after login
Question - What are Union and Intersection types?
Union :-
- Union types are used when the property can be more then one value, like string or number.
- For this reason they are also called OR and are used by using | symbol.
const names: readonly string[] = ["Rutvik", "Rohit", "Virat"];
names.push("Bumrah"); // Error: Property 'push' does not exist on type 'readonly string[]'.
Copy after login
Copy after login
Copy after login
Intersection :-
- Intersesction types are used when combines multiple types into one.
- For this reason they are also called AND and are used by using & symbol.
const numbers = [1, 2, 3]; // inferred to type number[]
numbers.push(4); // no error
Copy after login
Copy after login
Copy after login
Question - What are functions in Typescript ?
How to give the return type in function?
- We can give the return types of functions with : symbol after function name.
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
Copy after login
Copy after login
Copy after login
How to give type of parameters in function?
- We can give the type of parameters by mentioning them after each parameter with : symbol.
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
//No safety in indexes from 3
ourTuple.push('This is wrong');
Copy after login
Copy after login
Copy after login
How to give optional, default and rest parameters in function?
- With default parameter, we can mark a parameter as optional. Like this, where c is optional and denoted by ?.
let ourTuple: readonly [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
// throws error as it is readonly
ourTuple.push('Coding Hero took a day off');
Copy after login
Copy after login
Copy after login
- The default values(an ES6 feature), goes after the type.
interface CarTypes {
brand: string,
model: string,
year: number
}
const car: CarTypes = {
brand: "Tata",
model: "Punch",
year: 2020
};
Copy after login
Copy after login
Copy after login
- The rest parameters(an ES6 feature), are given the type of array, because they convert passed items in array.
interface CarTypes {
brand: string,
model: string,
year?: number
}
const car: CarTypes = {
brand: "Tata",
model: "Punch"
};
Copy after login
Copy after login
Question - What is casting in TypeScript?
- Casting is the process of overriding a type of a variable.
- Like in the below example, the type is unknown but is made string while using with the as keyword.
enum Direction {
Up = 1,
Down,
Left,
Right,
}
console.log(Direction.Up); // 1
console.log(Direction.Down); // 2
Copy after login
Copy after login
- We can also use <> in place of as. Both means the same.
let firstName: string = "Rutvik";
Copy after login
Copy after login
Copy after login
Question - What are Generics in TypeScript ?
- Generics in Typeascript allow you to create reusable components or dunctions that can work with multiple data types.
let age = 23;
Copy after login
Copy after login
Copy after login
Question - Utility Types in Typescript?
- TypeScript provides Utility Types to simplify common type transformations.
- These types make it easier to manipulate and interact with object and interface types.
- Here’s a breakdown of some commonly used utility types:
1. Partial
- Makes all properties of type T optional.
- Use case: When you want to create an object where only some properties are required.
let x: any = 10;
x = 'hello'; // No TypeScript error
console.log(x.toUpperCase()); // No TypeScript error
Copy after login
Copy after login
Copy after login
2. Required
- Makes all properties of type T required.
- Use case: When you want to enforce that all properties must be present.
let y: unknown = 10;
// Type assertion needed before using y as number
if (typeof y === 'number') {
console.log(y.toFixed(2));
}
Copy after login
Copy after login
Copy after login
3. Readonly
- Makes all properties of type T read-only.
- Use case: To ensure that an object’s properties cannot be modified.
function throwError(message: string): never {
throw new Error(message);
}
Copy after login
Copy after login
Copy after login
4. Pick
- Creates a type by picking a set of properties K from type T.
- Use case: When you need only specific properties from a type.
const names: string[] = ["Rutvik", "Rohit", "Virat"];
names.push("Bumrah"); // no error
Copy after login
Copy after login
Copy after login
5. Omit
- Creates a type by omitting a set of properties K from type T.
- Use case: When you want all properties except specific ones.
const names: readonly string[] = ["Rutvik", "Rohit", "Virat"];
names.push("Bumrah"); // Error: Property 'push' does not exist on type 'readonly string[]'.
Copy after login
Copy after login
Copy after login
6. Record
- Constructs a type with keys K and values of type T.
- Use case: To create an object type with fixed keys and consistent value types.
const numbers = [1, 2, 3]; // inferred to type number[]
numbers.push(4); // no error
Copy after login
Copy after login
Copy after login
7. Exclude
- Excludes from type T all types that are assignable to U.
- Use case: To filter out specific types.
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
Copy after login
Copy after login
Copy after login
8. Extract
- Extracts from type T only types that are assignable to U.
- Use case: To narrow down types to a specific subset.
let ourTuple: [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
//No safety in indexes from 3
ourTuple.push('This is wrong');
Copy after login
Copy after login
Copy after login
9. NonNullable
- Excludes null and undefined from type T.
- Use case: To ensure a value is neither null nor undefined.
let ourTuple: readonly [number, boolean, string];
// initialize correctly
ourTuple = [5, false, 'Coding Hero was here'];
// throws error as it is readonly
ourTuple.push('Coding Hero took a day off');
Copy after login
Copy after login
Copy after login
10. ReturnType
- Infers the return type of a function type.
- Use case: To capture and use the return type of a function.
interface CarTypes {
brand: string,
model: string,
year: number
}
const car: CarTypes = {
brand: "Tata",
model: "Punch",
year: 2020
};
Copy after login
Copy after login
Copy after login
11. InstanceType
- Constructs a type consisting of the instance type of a constructor function type T.
- Use case: To get the type of a class instance.
interface CarTypes {
brand: string,
model: string,
year?: number
}
const car: CarTypes = {
brand: "Tata",
model: "Punch"
};
Copy after login
Copy after login
12. Parameters
- Extracts the types of parameters of a function type.
- Use case: To reuse the parameter types of a function.
enum Direction {
Up = 1,
Down,
Left,
Right,
}
console.log(Direction.Up); // 1
console.log(Direction.Down); // 2
Copy after login
Copy after login
The above is the detailed content of TypeScript Interview Questions. For more information, please follow other related articles on the PHP Chinese website!