


How Should I Compare Floating-Point Numbers for Equality and Inequality?
Jan 06, 2025 am 01:09 AMDetermining Equality of Floating-Point Numbers Using Double.Epsilon
MSDN documentation suggests using a value greater than the Double.Epsilon constant for establishing the acceptable absolute margin of difference when determining whether two floating-point numbers can be considered equal. However, this recommendation raises some confusion.
Does Double.Epsilon Have Practical Use in Comparisons?
The MSDN wording has led to misunderstanding about the practical use of Double.Epsilon. As stated in the provided answer, Double.Epsilon represents the smallest representable non-denormal floating point value that isn't 0. It simply indicates the minimum size of a truncation error, not an acceptable margin for comparison.
Calculating a Suitable Epsilon for Equality Comparisons
For equality comparisons, a more practical approach is to use an epsilon based on the magnitude of the values being compared. For example:
public static bool AboutEqual(double x, double y) { double epsilon = Math.Max(Math.Abs(x), Math.Abs(y)) * 1E-15; return Math.Abs(x - y) <= epsilon; }
This method uses the larger of the absolute values of the two numbers multiplied by a small constant (1E-15) to determine the acceptable margin for comparison.
Considering Greater Than and Less Than Comparisons
For comparisons such as greater than or less than, the same principle applies. The epsilon should be calculated based on the magnitude of the numbers being compared to account for potential truncation errors.
Best Implementation for Equality and Inequality Comparisons
In summary, Double.Epsilon cannot be directly used as an acceptable margin for equality, greater than, less than, less than or equal to, or greater than or equal to comparisons for floating-point numbers. Instead, a context-specific epsilon should be calculated to ensure accurate comparisons.
The above is the detailed content of How Should I Compare Floating-Point Numbers for Equality and Inequality?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
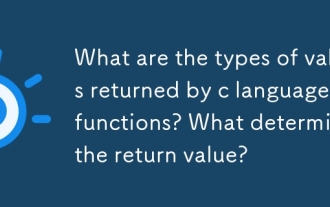
What are the types of values returned by c language functions? What determines the return value?
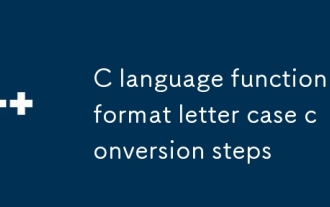
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
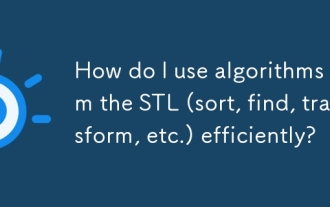
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
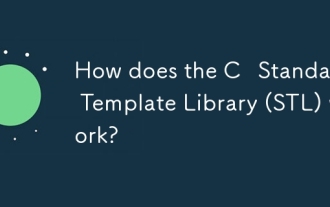
How does the C Standard Template Library (STL) work?
