


Introduction to @rxliuli/vista: A unified request interceptor library for both Fetch and XHR with middleware support.
@rxliuli/vista is a powerful homogeneous request interceptor library that supports unified interception of Fetch/XHR requests. It allows you to intervene at different stages of the request lifecycle, enabling various functions such as request monitoring, modification, and mocking.
Characteristics
- ? Supports both Fetch and XHR request interception
- ? Use middleware pattern, flexible and easy to expand
- ? Support interventions before and after requests
- ? Modifiable request and response data
- ? Zero dependency, compact size
- ? Supports browser environment only
Installation
1 2 3 4 5 |
|
Basic Usage
1 2 3 4 5 6 7 8 9 10 11 12 |
|
Advanced Use Cases
Add global request headers
1 2 3 4 5 6 |
|
Request Result Cache
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
Request failed, please retry
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
Dynamic modify response
1 2 3 4 5 6 7 8 9 10 |
|
API Reference
Vista Class
Main interceptor class, providing the following methods:
- use(middleware): Add middleware
- intercept(): Start intercepting requests
- destroy(): Stop intercepting requests
Middleware Context
The middleware function receives two parameters:
-
context: Contains request and response information
- req: Request object res: Response object
- type: Request type, fetch or xhr
- next: Call the function of the next middleware or original request
FAQ
- How to stop interception?
1 2 3 4 |
|
Does it support asynchronous operations?
Yes, the middleware supports async/await syntax.Does it support intercepting requests in Node.js?
No, it only supports intercepting requests in the browser.
Thanks
- xhook: A library that implements xhr interception, helpful for the implementation of some features.
- hono: An excellent web server framework that provides a lot of inspiration in its API.
Contribution Guidelines
Welcome to submit Issues and Pull Requests!
License
MIT License
Try it and tell me your experience, welcome any error or feature feedback.
The above is the detailed content of Introduction to @rxliuli/vista: A unified request interceptor library for both Fetch and XHR with middleware support.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
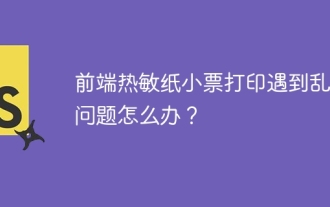
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
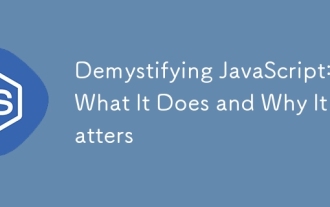
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
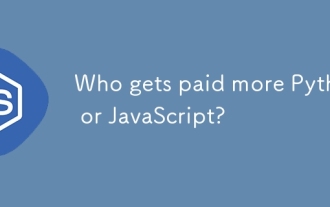
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
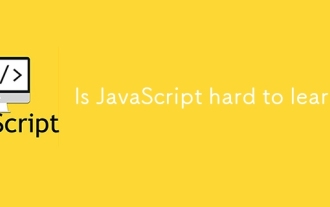
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
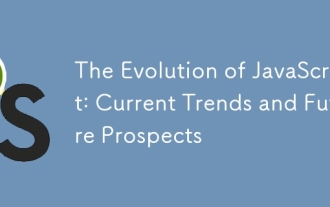
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
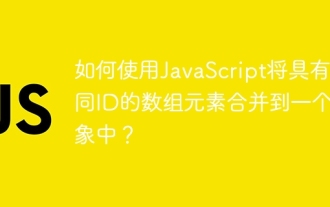
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
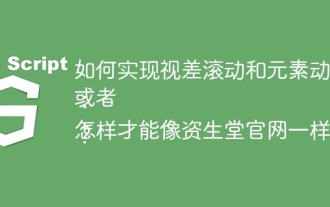
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
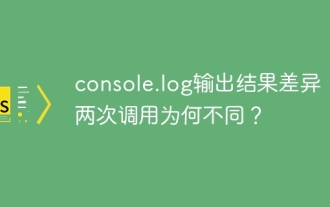
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
