


How to Integrate AI Models into Modern Web Applications: A Comprehensive Guide with Examples
Artificial Intelligence (AI) is no longer a buzzword—it’s a core component driving innovation in modern web applications. Integrating AI models into your web apps can bring advanced capabilities like natural language understanding, image recognition, and predictive analytics to life. This guide walks you through integrating AI models into your web application, complete with step-by-step examples and best practices.
1. Why Add AI to Web Applications?
AI can transform your web app by enabling:
- Enhanced User Experiences: From personalized recommendations to intelligent search.
- Automation: Powering chatbots, voice assistants, or workflow automation.
- Data-Driven Insights: Predictive analytics and real-time trend detection.
- Media Processing: Image recognition, audio transcription, and video analysis.
2. Choosing the Right AI Model
Before integrating AI, select the model that aligns with your app's needs. Popular AI categories include:
- Natural Language Processing (NLP): For text-based tasks like summarization, sentiment analysis, or chatbots. (e.g., OpenAI’s GPT APIs or Hugging Face Transformers)
- Image and Video Analysis: For object detection, face recognition, or video analysis. (e.g., TensorFlow.js or Google Vision API)
- Recommendation Engines: For personalized suggestions. (e.g., collaborative filtering models)
3. Example 1: Adding AI Chatbots Using GPT API
AI chatbots are a popular choice for automating customer service or powering virtual assistants.
Steps:
-
Backend Setup:
- Use Node.js and install the OpenAI SDK:
npm install openai
Copy after loginCopy after login
-
Configure the GPT API:
const { Configuration, OpenAIApi } = require('openai'); const configuration = new Configuration({ apiKey: process.env.OPENAI_API_KEY, }); const openai = new OpenAIApi(configuration); const generateResponse = async (prompt) => { const response = await openai.createCompletion({ model: "text-davinci-003", prompt: prompt, max_tokens: 150, }); return response.data.choices[0].text.trim(); };
Copy after loginCopy after login
-
Frontend Integration:
- Use React (or any frontend framework) to create a chatbot UI.
- Call the backend API endpoint to get AI-generated responses.
-
Real-Time Chat Enhancement:
- Integrate WebSocket for real-time interactions using libraries like Socket.IO.
4. Example 2: Image Recognition with TensorFlow.js
Integrate real-time image recognition into your app using TensorFlow.js.
Steps:
- Install TensorFlow.js:
npm install openai
-
Frontend Implementation:
- Load a pre-trained MobileNet model for image classification:
const { Configuration, OpenAIApi } = require('openai'); const configuration = new Configuration({ apiKey: process.env.OPENAI_API_KEY, }); const openai = new OpenAIApi(configuration); const generateResponse = async (prompt) => { const response = await openai.createCompletion({ model: "text-davinci-003", prompt: prompt, max_tokens: 150, }); return response.data.choices[0].text.trim(); };
Copy after loginCopy after login -
Interactive Image Upload:
- Create an interface for users to upload images.
- Display predictions directly on the UI.
5. Example 3: Building a Recommendation System
Use collaborative filtering or content-based filtering models to suggest items.
Steps:
-
Backend Model:
- Train a recommendation engine using Python (e.g., scikit-learn or TensorFlow).
- Deploy the model using Flask or FastAPI.
-
Integrate API in the Web App:
- Use Axios or Fetch to interact with the recommendation API:
npm install @tensorflow/tfjs @tensorflow-models/mobilenet
Copy after login -
Frontend Display:
- Render personalized recommendations dynamically based on user activity.
6. Example 4: Sentiment Analysis for Reviews or Feedback
Enhance your app by analyzing user sentiments in real time.
Steps:
- Install Hugging Face API:
import * as mobilenet from '@tensorflow-models/mobilenet'; import '@tensorflow/tfjs'; const classifyImage = async (imageElement) => { const model = await mobilenet.load(); const predictions = await model.classify(imageElement); console.log(predictions); };
-
Backend Implementation:
- Use the Hugging Face sentiment analysis model:
const fetchRecommendations = async (userId) => { const response = await fetch(`/api/recommendations/${userId}`); const recommendations = await response.json(); return recommendations; };
Copy after login -
Integrate Results in the UI:
- Highlight positive, negative, or neutral sentiments in feedback forms or dashboards.
7. Best Practices for Integrating AI
- Model Selection: Use pre-trained models when starting, then fine-tune for specific needs.
- Data Privacy: Ensure user data is anonymized and complies with regulations like GDPR.
- Performance Optimization: Use caching for repetitive requests and load models asynchronously.
- Scalability: Deploy AI services with containerization (e.g., Docker) and orchestration (e.g., Kubernetes).
Conclusion
Integrating AI into web applications can elevate user experiences and functionality, from chatbots to image recognition and personalized recommendations. By following these examples and best practices, you can seamlessly incorporate AI models into your projects and unlock the full potential of intelligent applications.
Call-to-Action
If you’ve integrated AI into your web apps or plan to, share your experiences in the comments! Have a specific use case in mind? Let’s discuss how AI can transform it. ?
The above is the detailed content of How to Integrate AI Models into Modern Web Applications: A Comprehensive Guide with Examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










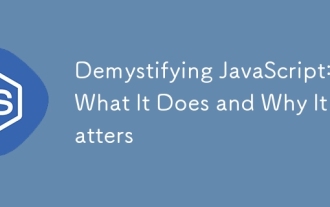
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
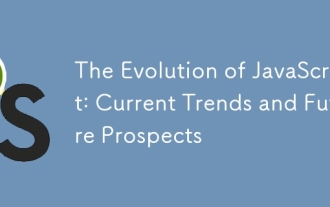
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
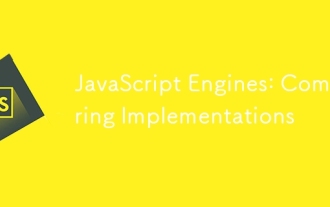
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
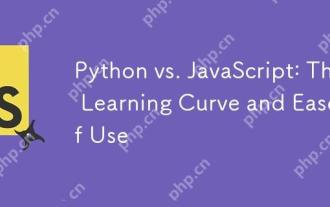
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
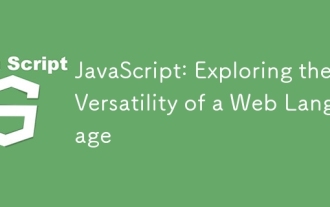
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
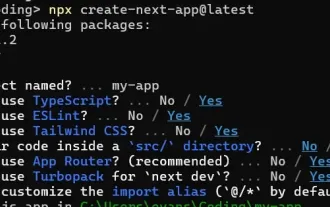
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
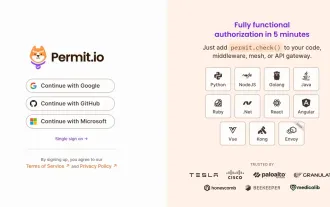
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
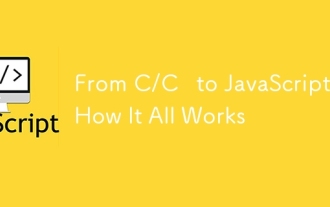
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
