Fundamentals of lambda expressions
Syntax Elements
Lambda Operator (->)
Divides the lambda expression into two parts:
Left side: Parameter list.
Right side: Lambda body (actions or return).
- Lambda Body Types:
Single expression: Directly returns the result of an expression.
Code block: Contains multiple instructions.
Examples of Lambda Expressions:
1) No parameters:
Example: () -> 98.6
Empty parameter list.
Returns a constant value 98.6 (type inferred as double).
Equivalent to method:
double myMeth() {
return 98.6;
}
Example: () -> Math.random() * 100
- Returns a pseudorandom number multiplied by 100.
2) With parameters:
Example: (n) -> 1.0 / n
Returns the reciprocal of n.
Parameter type usually inferred, but may be declared explicitly.
3) Return of Boolean values:
Example: (n) -> (n % 2) == 0
Returns true if n is even, false otherwise.
Simplified form (without parentheses in the parameter):
n -> (n % 2) == 0.
Considerations
The return type of a lambda expression is automatically inferred.
Parentheses in parameters are optional for lambda expressions with a single parameter.
The book suggests using parentheses for consistency in style.
General Summary
The lambda expression simplifies the creation of anonymous methods.
Flexible in terms of parameters and return types.
Adopts a concise syntax to improve code readability and expressiveness.
The above is the detailed content of Fundamentals of lambda expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


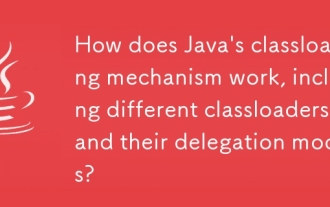
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
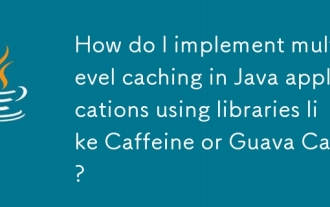
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
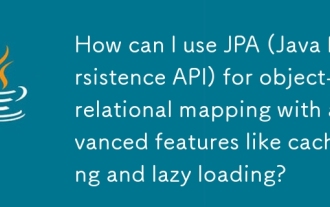
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
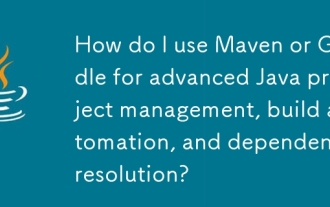
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
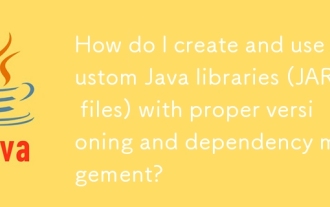
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
